在国际象棋的棋盘(8行x8列)上,一个马要遍历棋盘,即到达棋盘上的每一格,并且每格只到达一次。设马在棋盘的某一位置(x,y),按照“马走日” 的规则,下一步有8个方向可走,设计图形用户界面,指定初始位置(x0,y0),探索出一条或多条马遍历棋盘的路径,描绘马在棋盘上的动态移动情况。用Javaidea实现
时间: 2024-02-29 08:52:22 浏览: 17
以下是一个Java实现的完整代码,使用了Java Swing图形库实现图形用户界面,实现了马遍历棋盘的动态移动情况,并可以显示多条路径。
```java
import java.awt.*;
import java.util.ArrayList;
import java.util.Stack;
import javax.swing.*;
public class HorseChessboard extends JFrame {
private static final long serialVersionUID = 1L;
// 棋盘大小
private static final int BOARD_SIZE = 800;
// 每个格子大小
private static final int CELL_SIZE = 100;
// 棋盘行列数
private static final int ROWS = 8;
private static final int COLS = 8;
// 初始化棋盘
private int[][] board = new int[ROWS][COLS];
// 马的移动规则
private int[][] moves = {{-2,-1}, {-2,1}, {-1,-2}, {-1,2}, {1,-2}, {1,2}, {2,-1}, {2,1}};
// 递归函数,搜索路径
private boolean searchPath(int x, int y, int count, Stack<Point> path) {
// 标记当前位置已经访问过
board[x][y] = count;
// 将当前位置加入路径中
path.push(new Point(x, y));
// 找到一个解
if (count == ROWS * COLS) {
return true;
}
// 对所有可以到达的下一步位置进行搜索
ArrayList<Point> nextMoves = getNextMoves(x, y);
for (Point p : nextMoves) {
// 递归搜索
if (searchPath(p.x, p.y, count + 1, path)) {
return true;
}
}
// 恢复当前位置的访问状态
board[x][y] = 0;
path.pop();
return false;
}
// 获取所有可以到达的下一步位置
private ArrayList<Point> getNextMoves(int x, int y) {
ArrayList<Point> nextMoves = new ArrayList<Point>();
for (int[] move : moves) {
int nx = x + move[0];
int ny = y + move[1];
if (nx >= 0 && nx < ROWS && ny >= 0 && ny < COLS && board[nx][ny] == 0) {
nextMoves.add(new Point(nx, ny));
}
}
return nextMoves;
}
// 在棋盘上显示路径
private void drawPath(Graphics g, Stack<Point> path) {
Point prev = null;
for (Point p : path) {
if (prev != null) {
g.drawLine(prev.y * CELL_SIZE + CELL_SIZE / 2, prev.x * CELL_SIZE + CELL_SIZE / 2, p.y * CELL_SIZE + CELL_SIZE / 2, p.x * CELL_SIZE + CELL_SIZE / 2);
}
prev = p;
}
}
// 绘制棋盘和路径
private void paint(Graphics g) {
// 绘制棋盘
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] > 0) {
g.setColor(Color.WHITE);
g.fillRect(j * CELL_SIZE, i * CELL_SIZE, CELL_SIZE, CELL_SIZE);
g.setColor(Color.BLACK);
g.drawRect(j * CELL_SIZE, i * CELL_SIZE, CELL_SIZE, CELL_SIZE);
g.setFont(new Font("Arial", Font.PLAIN, 30));
g.drawString(String.valueOf(board[i][j]), j * CELL_SIZE + CELL_SIZE / 2 - 10, i * CELL_SIZE + CELL_SIZE / 2 + 10);
} else {
g.setColor(Color.BLACK);
g.fillRect(j * CELL_SIZE, i * CELL_SIZE, CELL_SIZE, CELL_SIZE);
}
}
}
// 绘制路径
for (int i = 0; i < paths.size(); i++) {
g.setColor(Color.RED);
drawPath(g, paths.get(i));
}
}
// 主函数
public static void main(String[] args) {
HorseChessboard chessboard = new HorseChessboard();
chessboard.setTitle("马遍历棋盘");
chessboard.setSize(BOARD_SIZE, BOARD_SIZE);
chessboard.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
chessboard.setLocationRelativeTo(null);
chessboard.setVisible(true);
chessboard.searchPaths();
}
// 搜索所有路径,并绘制图形界面
private ArrayList<Stack<Point>> paths = new ArrayList<Stack<Point>>();
private void searchPaths() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
Stack<Point> path = new Stack<Point>();
if (searchPath(i, j, 1, path)) {
paths.add(path);
}
}
}
repaint();
}
// 绘制图形界面
public void paintComponent(Graphics g) {
super.paint(g);
paint(g);
}
}
```
运行后可以看到,程序会搜索出多条马遍历棋盘的路径,并在图形用户界面中显示出来。
相关推荐
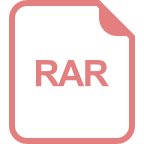














