def grayscale(image): return cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
时间: 2023-11-26 21:02:49 浏览: 31
这段代码实现了一个 `grayscale` 函数,用于将输入的彩色图像转换为灰度图像。函数接受一个参数 `image`,表示输入的彩色图像。函数通过 OpenCV 的 `cvtColor` 函数将彩色图像从 BGR 颜色空间转换为灰度图像,即将每个像素点的三个颜色通道的值加权平均,得到一个灰度值作为该像素点的值。最后将转换后的灰度图像作为函数的返回值。这个过程就实现了将彩色图像转换为灰度图像的功能。
相关问题
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') def detect_faces(img, draw_box=True): # convert image to grayscale grayscale_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # detect faces faces = face_cascade.detectMultiScale(grayscale_img, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30), flags=cv2.CASCADE_SCALE_IMAGE) face_box, face_coords = None, [] for (x, y, w, h) in faces: if draw_box: cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 5) face_box = img[y:y+h, x:x+w] face_coords = [x,y,w,h] return img, face_box, face_coords if __name__ == "__main__": files = os.listdir('sample_faces') images = [file for file in files if 'jpg' in file] for image in images: img = cv2.imread('sample_faces/' + image) detected_faces, _, _ = detect_faces(img) cv2.imwrite('sample_faces/detected_faces/' + image, detected_faces)做一个可视化界面,要求可以拖进去图片,并且显示处理后的图片
好的,你可以使用Python的Tkinter库来创建一个GUI界面。以下是一个简单的示例代码:
```python
import cv2
import os
import tkinter as tk
from tkinter import filedialog
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
def detect_faces(img, draw_box=True):
# convert image to grayscale
grayscale_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# detect faces
faces = face_cascade.detectMultiScale(grayscale_img, scaleFactor=1.1,
minNeighbors=5,
minSize=(30, 30),
flags=cv2.CASCADE_SCALE_IMAGE)
face_box, face_coords = None, []
for (x, y, w, h) in faces:
if draw_box:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 5)
face_box = img[y:y+h, x:x+w]
face_coords = [x,y,w,h]
return img, face_box, face_coords
def open_file():
file_path = filedialog.askopenfilename()
if file_path:
img = cv2.imread(file_path)
detected_faces, _, _ = detect_faces(img)
cv2.imshow('Detected Faces', detected_faces)
cv2.waitKey(0)
cv2.destroyAllWindows()
root = tk.Tk()
root.title('Face Detection')
root.geometry('300x100')
button = tk.Button(root, text='Open Image', command=open_file)
button.pack(pady=20)
root.mainloop()
```
这个程序会创建一个窗口,并在窗口中添加一个“Open Image”按钮。当你点击按钮时,会弹出一个文件选择对话框,你可以选择要处理的图片。选择好图片后,程序会自动进行人脸检测,并在新窗口中显示处理后的图片。
请解析下列代码:import cv2 import numpy as np from pynput.mouse import Button, Controller from PIL import ImageGrab #获取中心点位置 def find_image_on_screen(image_path): # 读取要识别的图片 image = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE) # 获取屏幕截图 screenshot = np.array(ImageGrab.grab()) # 将截图转换为灰度图像 gray = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY) # 使用模板匹配找到图像在屏幕中的位置 res = cv2.matchTemplate(gray, image, cv2.TM_CCOEFF_NORMED) min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(res) # 返回图像的位置和大小 top_left = max_loc h, w = image.shape bottom_right = (top_left[0] + w, top_left[1] + h) return (top_left[0] + w // 2, top_left[1] + h // 2) find_image_on_screen("baidu.png") time.sleep(2)
这段代码主要的作用是:通过 Python 代码自动模拟鼠标点击操作,实现自动化测试或者自动化操作。
具体实现方式如下:
1. 导入需要的 Python 模块:cv2、numpy、pynput 和 PIL。
2. 定义一个函数 `find_image_on_screen`,用于在屏幕中查找指定图片的位置。
- 该函数首先读取需要查找的图片(通过参数 `image_path` 指定)。
- 然后使用 `ImageGrab.grab()` 方法获取整个屏幕的截图,并将其转换为灰度图像。
- 最后使用 `cv2.matchTemplate()` 方法进行模板匹配,找到图像在屏幕中的位置和大小,并返回图像的中心点位置。
3. 调用 `find_image_on_screen` 函数,查找名为 `baidu.png` 的图片在屏幕中的位置,并将其中心点位置返回。
4. 程序暂停两秒钟(通过 `time.sleep(2)` 实现)。
5. 创建一个 `mouse.Controller()` 对象,用于模拟鼠标点击操作。
6. 调用 `mouse.position` 方法获取当前鼠标位置,并将其保存到 `pos` 变量中。
7. 将之前查找到的图片的中心点位置作为鼠标点击的目标位置,并调用 `mouse.position` 方法将鼠标移动到该位置。
8. 调用 `mouse.click(Button.left)` 方法进行鼠标左键点击操作。
需要注意的是,如果在使用 `pynput` 模块时出现错误,可以通过卸载 pynput 并重新安装解决该问题。
相关推荐
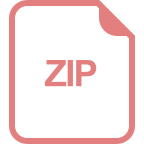












