基类Point派生出一个Circle(圆)类,Circle(圆)类再派生出一个Cylinder(圆柱体)类。 基类Point的有数据成员x,y,若干成员函数用于对数据成的操作; Circle(圆)类增加数据成员r(半径),以及求面积的成员函数area,和若干需要的成员函数; Cylinder(圆柱体)类,再增加数据成员h(高),以及求圆柱表面积的成员函数area和求圆柱体积的成员函数volume,及若干需要的成员函数; 每个类都有若干构造函数及析构函数。设计main函数完成测试。
时间: 2023-06-19 22:05:46 浏览: 147
好的,这是您的第一个问题。
要基于Point类派生Circle类和Cylinder类,您可以使用如下的代码:
```c++
#include <iostream>
using namespace std;
class Point
{
private:
double x, y;
public:
Point(double x0 = 0, double y0 = 0)
{
x = x0;
y = y0;
}
Point(const Point& p)
{
x = p.x;
y = p.y;
}
void set(double x0, double y0)
{
x = x0;
y = y0;
}
double getX() const
{
return x;
}
double getY() const
{
return y;
}
};
class Circle : public Point
{
private:
double r;
public:
Circle(double x0 = 0, double y0 = 0, double r0 = 0) : Point(x0, y0)
{
r = r0;
}
Circle(const Circle& c)
{
set(c.getX(), c.getY());
r = c.r;
}
void setRadius(double r0)
{
r = r0;
}
double getRadius() const
{
return r;
}
double area() const
{
return 3.1415 * r * r;
}
};
class Cylinder : public Circle
{
private:
double h;
public:
Cylinder(double x0 = 0, double y0 = 0, double r0 = 0, double h0 = 0) : Circle(x0, y0, r0)
{
h = h0;
}
Cylinder(const Cylinder& c)
{
set(c.getX(), c.getY());
setRadius(c.getRadius());
h = c.h;
}
void setHeight(double h0)
{
h = h0;
}
double getHeight() const
{
return h;
}
double area() const
{
return 2 * Circle::area() + 2 * 3.1415 * getRadius() * h;
}
double volume() const
{
return Circle::area() * h;
}
};
int main()
{
Cylinder c(1, 2, 3, 4);
cout << "Radius: " << c.getRadius() << endl;
cout << "Height: " << c.getHeight() << endl;
cout << "Area: " << c.area() << endl;
cout << "Volume: " << c.volume() << endl;
return 0;
}
```
在这个示例代码中,我们首先定义了一个Point类,然后分别派生出Circle类和Cylinder类。在派生的过程中,我们使用了public关键字,表示派生类可以访问基类的公有成员。
在Circle类中,我们增加了数据成员r(半径),并且实现了计算面积的函数area。在Cylinder类中,我们再增加了数据成员h(高),并且实现了计算圆柱体表面积的函数area和计算圆柱体积的函数volume。
在测试中,我们创建了一个Cylinder对象,并分别输出了它的半径、高、表面积和体积。我们可以看到,当我们调用area函数时,由于派生类中有与基类同名的函数area,我们需要使用作用域解析符“::”来明确指定调用基类的area函数。
阅读全文
相关推荐
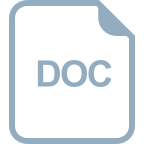
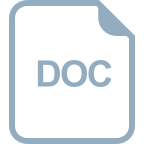
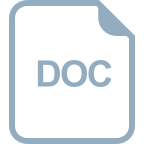









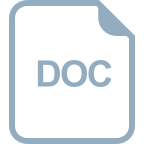
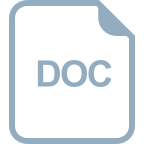
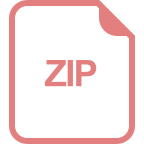
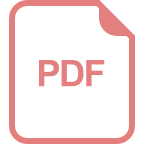
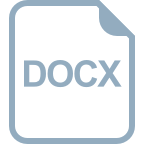