java怎么实现c++的isalpha()
时间: 2023-05-20 15:05:03 浏览: 103
Java中可以使用Character类的isLetter()方法来实现C语言中的isalpha()函数。isLetter()方法用于判断一个字符是否为字母,如果是字母则返回true,否则返回false。以下是一个示例代码:
```java
public class Main {
public static void main(String[] args) {
char c = 'A';
if (Character.isLetter(c)) {
System.out.println(c + " is an alphabet.");
} else {
System.out.println(c + " is not an alphabet.");
}
}
}
```
输出结果为:
```
A is an alphabet.
```
相关问题
java isalpha
在Java中,没有isalpha函数。isalpha是C/C++语言中的一个字符处理函数,用于判断一个字符是否为字母。而在Java中,可以使用Character类中的isLetter方法来判断一个字符是否为字母。
isLetter方法是一个boolean类型的方法,它的作用是判断一个字符是否为字母。如果是字母,则返回true,否则返回false。示例如下:
```java
char ch = 'A';
if (Character.isLetter(ch)) {
System.out.println(ch + "是字母");
} else {
System.out.println(ch + "不是字母");
}
```
用c++/java、python编程实现一种加密算法,能完成加密和解密工作。
在C++中,我们可以使用简单的Caesar Cipher(凯撒密码)作为示例,这是一种替换加密技术,通过将明文字符按照字母表位移固定距离进行加密。下面是一个基础版本的C++实现:
```cpp
#include <iostream>
#include <string>
std::string encryptCaesar(const std::string& plaintext, int shift) {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) {
c = islower(c) ? 'a' + ((c - 'a' + shift) % 26) : 'A' + ((c - 'A' + shift) % 26);
}
ciphertext += c;
}
return ciphertext;
}
std::string decryptCaesar(const std::string& ciphertext, int shift) {
return encryptCaesar(ciphertext, -shift);
}
int main() {
std::string message = "Hello, World!";
int shift = 3; // 示例位移值
std::string encrypted = encryptCaesar(message, shift);
std::cout << "Encrypted: " << encrypted << std::endl;
std::string decrypted = decryptCaesar(encrypted, shift);
std::cout << "Decrypted: " << decrypted << std::endl;
return 0;
}
```
在Python中,同样可以编写凯撒密码的实现:
```python
def caesar_encrypt(text, shift):
encrypted_text = ""
for char in text:
if char.isalpha():
shift_amount = shift % 26
if char.islower():
encrypted_text += chr((ord(char) - ord('a') + shift_amount) % 26 + ord('a'))
else:
encrypted_text += chr((ord(char) - ord('A') + shift_amount) % 26 + ord('A'))
else:
encrypted_text += char
return encrypted_text
def caesar_decrypt(text, shift):
return caesar_encrypt(text, -shift)
message = "Hello, World!"
shift = 3
encrypted = caesar_encrypt(message, shift)
print("Encrypted:", encrypted)
decrypted = caesar_decrypt(encrypted, shift)
print("Decrypted:", decrypted)
```
这两个例子展示了如何使用C++和Python实现基本的凯撒密码加密和解密功能。
阅读全文
相关推荐
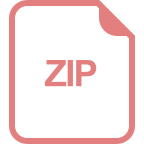
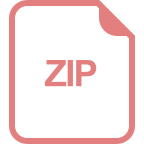
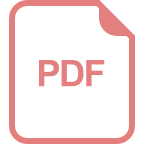

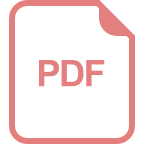
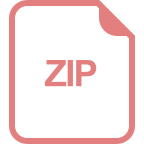
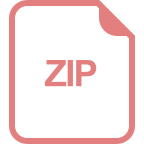
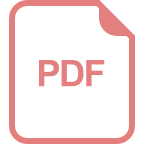
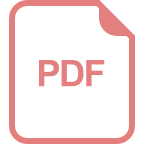
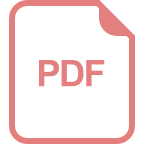