C# AutoCAD2010选择dwg中的一个块,获取其位置后,再在电脑本地选择一个外部块,在该位置去替换它 ,完整代码
时间: 2024-03-11 15:50:36 浏览: 96
以下是一个示例代码,实现了在 AutoCAD 中选择一个块,并在本地选择一个块文件,将其替换到选择的块的位置:
```csharp
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.EditorInput;
using Autodesk.AutoCAD.Geometry;
using Autodesk.AutoCAD.Runtime;
using System.IO;
using System.Windows.Forms;
namespace ReplaceBlock
{
public class Class1
{
[CommandMethod("ReplaceBlock")]
public void ReplaceBlock()
{
// 获取当前文档和数据库
Document acDoc = Application.DocumentManager.MdiActiveDocument;
Database acCurDb = acDoc.Database;
// 提示用户选择要替换的块
PromptEntityOptions peo = new PromptEntityOptions("\n选择要替换的块:");
peo.SetRejectMessage("\n请选择块实体。");
peo.AddAllowedClass(typeof(BlockReference), true);
PromptEntityResult per = acDoc.Editor.GetEntity(peo);
if (per.Status != PromptStatus.OK)
{
return;
}
// 获取选中的块的位置和属性
using (Transaction acTrans = acCurDb.TransactionManager.StartTransaction())
{
BlockReference br = acTrans.GetObject(per.ObjectId, OpenMode.ForRead) as BlockReference;
if (br == null)
{
return;
}
Point3d position = br.Position;
ObjectId blockId = br.BlockTableRecord;
BlockTableRecord btr = acTrans.GetObject(blockId, OpenMode.ForRead) as BlockTableRecord;
if (btr == null)
{
return;
}
// 将块分解为实体,并获取属性信息
BlockTableRecord modelSpace = acTrans.GetObject(acCurDb.CurrentSpaceId, OpenMode.ForWrite) as BlockTableRecord;
ObjectIdCollection ids = new ObjectIdCollection();
foreach (ObjectId objId in btr)
{
ids.Add(objId);
}
DBObjectCollection objs = new DBObjectCollection();
acCurDb.Explode(ids, objs);
AttributeCollection attrs = null;
foreach (DBObject obj in objs)
{
Entity ent = obj as Entity;
if (ent != null)
{
modelSpace.AppendEntity(ent);
acTrans.AddNewlyCreatedDBObject(ent, true);
if (ent is BlockReference)
{
BlockReference blkRef = ent as BlockReference;
if (blkRef.BlockTableRecord == blockId)
{
attrs = blkRef.AttributeCollection;
}
}
}
}
// 提示用户选择要替换的块文件
OpenFileDialog ofd = new OpenFileDialog();
ofd.Filter = "DWG文件|*.dwg";
ofd.Multiselect = false;
if (ofd.ShowDialog() != DialogResult.OK)
{
return;
}
// 在选择的块的位置创建新的块,并设置属性
BlockTable bt = acTrans.GetObject(acCurDb.BlockTableId, OpenMode.ForRead) as BlockTable;
if (bt == null)
{
return;
}
BlockTableRecord btrNew = new BlockTableRecord();
btrNew.Name = "*U";
bt.UpgradeOpen();
bt.Add(btrNew);
acTrans.AddNewlyCreatedDBObject(btrNew, true);
BlockReference brNew = new BlockReference(position, btrNew.ObjectId);
modelSpace.AppendEntity(brNew);
acTrans.AddNewlyCreatedDBObject(brNew, true);
if (attrs != null)
{
foreach (ObjectId attrId in attrs)
{
AttributeReference attr = acTrans.GetObject(attrId, OpenMode.ForRead) as AttributeReference;
if (attr != null)
{
AttributeReference attrNew = attr.Clone() as AttributeReference;
if (attrNew != null)
{
brNew.AttributeCollection.AppendAttribute(attrNew);
acTrans.AddNewlyCreatedDBObject(attrNew, true);
}
}
}
}
// 将选择的块插入到新创建的块的位置
Database acDbExt = new Database(false, true);
acDbExt.ReadDwgFile(ofd.FileName, FileOpenMode.OpenForReadAndAllShare, false, "");
using (Transaction acTransExt = acDbExt.TransactionManager.StartTransaction())
{
BlockTable btExt = acTransExt.GetObject(acDbExt.BlockTableId, OpenMode.ForRead) as BlockTable;
if (btExt == null)
{
return;
}
BlockTableRecord btrExt = acTransExt.GetObject(btExt[BlockTableRecord.ModelSpace], OpenMode.ForRead) as BlockTableRecord;
if (btrExt == null)
{
return;
}
foreach (ObjectId objId in btrExt)
{
Entity entExt = acTransExt.GetObject(objId, OpenMode.ForRead) as Entity;
if (entExt != null && entExt is BlockReference)
{
BlockReference blkRefExt = entExt as BlockReference;
if (blkRefExt.Name == Path.GetFileNameWithoutExtension(ofd.FileName))
{
BlockReference blkRefExtNew = blkRefExt.Clone() as BlockReference;
if (blkRefExtNew != null)
{
blkRefExtNew.Position = position;
brNew.SetBlockReferenceId(blkRefExtNew.BlockTableRecord, true);
foreach (ObjectId attrId in blkRefExt.AttributeCollection)
{
AttributeReference attrExt = acTransExt.GetObject(attrId, OpenMode.ForRead) as AttributeReference;
if (attrExt != null)
{
AttributeReference attrNew = attrExt.Clone() as AttributeReference;
if (attrNew != null)
{
blkRefExtNew.AttributeCollection.AppendAttribute(attrNew);
acTrans.AddNewlyCreatedDBObject(attrNew, true);
}
}
}
break;
}
}
}
}
acTransExt.Commit();
}
// 删除原来的块
br.Erase();
// 保存修改后的图形
acTrans.Commit();
}
}
}
}
```
请注意,此示例代码仅供参考,实际使用时需要根据具体情况进行修改和调整。同时,为了使代码更易于理解和维护,建议将其分解为多个方法或类。
阅读全文
相关推荐
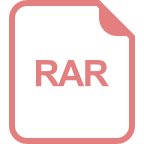

















