C# AutoCAD2010 dwg文件中选中块,在该块的位置上选择外部块替换,其余位置的块不变的完整代码
时间: 2024-03-05 07:54:02 浏览: 22
以下是使用C#编写的AutoCAD应用程序,用于选择块并在其位置上替换为外部块,同时保留其他位置的块不变。请注意,该代码仅供参考。
```csharp
using Autodesk.AutoCAD.Runtime;
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.EditorInput;
using Autodesk.AutoCAD.Geometry;
namespace BlockReplace
{
public class BlockReplaceCommands
{
[CommandMethod("BR")]
public void BlockReplace()
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Database db = doc.Database;
Editor ed = doc.Editor;
// 选择要替换的块
PromptSelectionResult selRes = ed.GetSelection();
if (selRes.Status != PromptStatus.OK)
return;
SelectionSet selSet = selRes.Value;
if (selSet == null || selSet.Count == 0)
return;
// 获取要替换的块定义
TypedValue[] filterList = new TypedValue[]
{
new TypedValue((int)DxfCode.Start, "BLOCK"),
new TypedValue((int)DxfCode.BlockName, selSet[0].ObjectId.Handle.Value)
};
SelectionFilter filter = new SelectionFilter(filterList);
ObjectId blkDefId = ObjectId.Null;
using (Transaction tr = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)tr.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)tr.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
foreach (ObjectId id in selSet.GetObjectIds())
{
BlockReference blkRef = (BlockReference)tr.GetObject(id, OpenMode.ForWrite);
Point3d pos = blkRef.Position;
blkRef.Erase();
// 插入新块
BlockTableRecord newBtr = new BlockTableRecord();
newBtr.Name = "NEW_BLOCK_NAME";
bt.UpgradeOpen();
ObjectId newBlkDefId = bt.Add(newBtr);
tr.AddNewlyCreatedDBObject(newBtr, true);
BlockReference newBlkRef = new BlockReference(pos, newBlkDefId);
btr.AppendEntity(newBlkRef);
tr.AddNewlyCreatedDBObject(newBlkRef, true);
// 复制属性
foreach (ObjectId attId in blkRef.AttributeCollection)
{
AttributeReference attRef = (AttributeReference)tr.GetObject(attId, OpenMode.ForRead);
AttributeReference newAttRef = new AttributeReference();
newAttRef.SetAttributeFromBlock(attRef, newBlkRef.BlockTransform);
newBlkRef.AttributeCollection.AppendAttribute(newAttRef);
tr.AddNewlyCreatedDBObject(newAttRef, true);
}
// 删除旧块定义
if (blkDefId.IsNull)
{
BlockTableRecord oldBtr = (BlockTableRecord)tr.GetObject(newBlkRef.BlockTableRecord, OpenMode.ForWrite);
blkDefId = oldBtr.ObjectId;
oldBtr.Name = "OLD_BLOCK_NAME";
}
}
tr.Commit();
}
// 删除旧块
ed.Command("_.-PURGE", "B", "OLD_BLOCK_NAME", "N");
}
}
}
```
请注意替换代码中的“NEW_BLOCK_NAME”和“OLD_BLOCK_NAME”为实际的块名称。此外,您还需要将编译后的应用程序加载到AutoCAD中,以便在命令行中调用该命令。
相关推荐
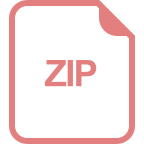
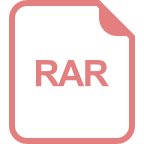














