C# AutoCAD2010选择dwg中的一个块,获取其位置后,再在电脑本地选择一个外部块,在该位置去替换它,完整代码
时间: 2024-03-10 15:43:31 浏览: 110
好的,以下是完整代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Autodesk.AutoCAD.Runtime;
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.EditorInput;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.Geometry;
using System.IO;
namespace ReplaceBlock
{
public class Commands
{
[CommandMethod("RB")]
public void ReplaceBlock()
{
Document doc = Application.DocumentManager.MdiActiveDocument;
Editor ed = doc.Editor;
// 选择第一个块
PromptEntityOptions peo1 = new PromptEntityOptions("\n选择第一个块: ");
peo1.SetRejectMessage("\n必须选择块对象.");
peo1.AddAllowedClass(typeof(BlockReference), true);
PromptEntityResult per1 = ed.GetEntity(peo1);
if (per1.Status != PromptStatus.OK)
{
ed.WriteMessage("\n无效的选择.");
return;
}
// 获取第一个块的位置
Transaction tr1 = doc.TransactionManager.StartTransaction();
using (tr1)
{
BlockReference br1 = tr1.GetObject(per1.ObjectId, OpenMode.ForRead) as BlockReference;
if (br1 != null)
{
Point3d pt1 = br1.Position;
// 选择外部块文件
PromptOpenFileOptions pfo = new PromptOpenFileOptions("\n选择要插入的块文件: ");
pfo.Filter = "AutoCAD 块文件 (*.dwg)|*.dwg";
PromptFileNameResult pfnr = ed.GetFileNameForOpen(pfo);
if (pfnr.Status != PromptStatus.OK)
{
ed.WriteMessage("\n必须选择有效的块文件.");
return;
}
// 加载块文件并获取块定义
Database db2 = new Database(false, true);
using (db2)
{
db2.ReadDwgFile(pfnr.StringResult, FileOpenMode.OpenForReadAndAllShare, false, null);
ObjectIdCollection ids = new ObjectIdCollection();
foreach (ObjectId id in db2.BlockTableId)
{
ids.Add(id);
}
PromptEntityOptions peo2 = new PromptEntityOptions("\n选择要插入的块: ");
peo2.SetRejectMessage("\n必须选择块对象.");
peo2.AddAllowedClass(typeof(BlockReference), true);
peo2.AddAllowedClass(typeof(BlockTableRecord), true);
peo2.AddAllowedClass(typeof(DynamicBlockReference), true);
PromptEntityResult per2 = ed.GetEntity(peo2);
if (per2.Status != PromptStatus.OK)
{
ed.WriteMessage("\n无效的选择.");
return;
}
Entity ent2 = tr1.GetObject(per2.ObjectId, OpenMode.ForRead) as Entity;
if (ent2 is BlockTableRecord)
{
BlockTableRecord btr2 = ent2 as BlockTableRecord;
foreach (ObjectId id in btr2)
{
ids.Add(id);
}
}
else if (ent2 is BlockReference)
{
BlockReference br2 = ent2 as BlockReference;
ids.Add(br2.BlockTableRecord);
}
// 引用块并插入到块表记录中
BlockTableRecord btr = (BlockTableRecord)tr1.GetObject(br1.BlockTableRecord, OpenMode.ForWrite);
BlockTable bt = (BlockTable)tr1.GetObject(btr.Database.BlockTableId, OpenMode.ForRead);
ObjectId idBtr = ObjectId.Null;
foreach (ObjectId id in ids)
{
if (bt.Has(id))
{
BlockTableRecord btr2 = (BlockTableRecord)tr1.GetObject(id, OpenMode.ForRead);
if (!btr2.IsLayout && !btr2.IsAnonymous)
{
idBtr = id;
break;
}
}
}
if (idBtr.IsNull)
{
ed.WriteMessage("\n无法找到有效的块.");
return;
}
BlockReference br = new BlockReference(pt1, idBtr);
btr.AppendEntity(br);
tr1.AddNewlyCreatedDBObject(br, true);
// 复制块属性
BlockTableRecord btrSrc = (BlockTableRecord)tr1.GetObject(idBtr, OpenMode.ForRead);
BlockTableRecord btrDest = (BlockTableRecord)tr1.GetObject(br.BlockTableRecord, OpenMode.ForWrite);
foreach (ObjectId id in btrSrc)
{
Entity ent = tr1.GetObject(id, OpenMode.ForRead) as Entity;
if (ent != null)
{
Entity entCopy = ent.Clone() as Entity;
if (entCopy != null)
{
btrDest.AppendEntity(entCopy);
tr1.AddNewlyCreatedDBObject(entCopy, true);
}
}
}
// 删除第一个块
br1.Erase();
}
}
tr1.Commit();
}
}
}
}
```
请注意,此代码仅适用于 AutoCAD 2010 并使用 C# 语言。使用时请将其编译为 .dll 文件并加载到 AutoCAD 中。在命令行中输入“RB”即可执行命令。
阅读全文
相关推荐
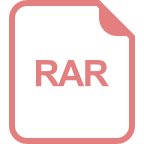

















