edges = cv2.Canny(img, threshold1=30, threshold2=100) cv2.imshow('Edges', edges) cv2.waitKey(0)
时间: 2024-05-17 12:18:00 浏览: 18
这段代码是使用OpenCV库中的Canny边缘检测算法来检测图像中的边缘,并将结果显示在名为"Edges"的窗口中。其中,threshold1和threshold2是Canny算法中的两个阈值参数,用于控制边缘检测的敏感度。cv2.imshow()函数用于显示图像,cv2.waitKey(0)函数用于等待用户按下任意键后关闭窗口。
相关问题
import cv2 import numpy as np # 加载图像 img = cv2.imread('color_blind_road_2.png') # 转换为灰度图像 gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # 直线检测 edges = cv2.Canny(gray, 50, 150, apertureSize=3) lines = cv2.HoughLinesP(edges, 1, np.pi/180, threshold=100, minLineLength=100, maxLineGap=10) for line in lines: x1, y1, x2, y2 = line[0] cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2) # 阈值分割 ret, thresh = cv2.threshold(gray, 150, 255, cv2.THRESH_BINARY) thresh = cv2.medianBlur(thresh, 5) # 彩色连续性空间分割 hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV) h, s, v = cv2.split(hsv) mask = cv2.inRange(h, 0, 20) | cv2.inRange(h, 160, 180) mask = cv2.bitwise_and(thresh, mask) # 纹理分割 gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) texture = cv2.Canny(gray, 100, 200, apertureSize=3) texture = cv2.dilate(texture, None, iterations=3) texture = cv2.erode(texture, None, iterations=3) texture = cv2.bitwise_and(thresh, texture) # 显示结果 cv2.imshow('img', img) cv2.imshow('thresh', thresh) cv2.imshow('color', mask) cv2.imshow('texture', texture) cv2.waitKey(0) cv2.destroyAllWindows()改进代码
以下是对代码的一些改进建议:
1. 在 import 语句之间添加空格,使代码更易于阅读。
2. 使用函数封装代码,将不同的操作分别放入不同的函数中,可以使代码更加清晰和易于维护。
3. 添加异常处理,以避免因为文件路径或读取失败而导致程序崩溃。
4. 将一些常量或者需要调整的参数提取出来,以方便后续调整和修改。
5. 添加注释,以便代码的阅读和理解。
6. 使用 with 语句释放资源。
改进后的代码如下:
```python
import cv2
import numpy as np
def load_image(path):
"""加载图片"""
try:
img = cv2.imread(path)
return img
except Exception as e:
print(e)
return None
def gray_transform(img):
"""灰度转换"""
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
return gray
def edge_detection(img, threshold1=50, threshold2=150, apertureSize=3):
"""边缘检测"""
edges = cv2.Canny(img, threshold1, threshold2, apertureSize=apertureSize)
return edges
def line_detection(img, edges, threshold=100, minLineLength=100, maxLineGap=10):
"""直线检测"""
lines = cv2.HoughLinesP(edges, 1, np.pi/180, threshold=threshold, minLineLength=minLineLength, maxLineGap=maxLineGap)
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
return img
def threshold_segmentation(img, threshold=150):
"""阈值分割"""
ret, thresh = cv2.threshold(img, threshold, 255, cv2.THRESH_BINARY)
thresh = cv2.medianBlur(thresh, 5)
return thresh
def hsv_segmentation(img, lower_range, upper_range):
"""HSV颜色空间分割"""
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
mask = cv2.inRange(hsv, lower_range, upper_range)
return mask
def color_segmentation(img, thresh, lower_range1=(0, 100, 100), upper_range1=(20, 255, 255), lower_range2=(160, 100, 100), upper_range2=(180, 255, 255)):
"""颜色分割"""
mask1 = hsv_segmentation(img, lower_range1, upper_range1)
mask2 = hsv_segmentation(img, lower_range2, upper_range2)
mask = cv2.bitwise_or(mask1, mask2)
mask = cv2.bitwise_and(thresh, mask)
return mask
def texture_segmentation(img, thresh, threshold1=100, threshold2=200, iterations=3):
"""纹理分割"""
gray = gray_transform(img)
texture = cv2.Canny(gray, threshold1, threshold2, apertureSize=3)
texture = cv2.dilate(texture, None, iterations=iterations)
texture = cv2.erode(texture, None, iterations=iterations)
texture = cv2.bitwise_and(thresh, texture)
return texture
def show_image(img, winname='image'):
"""显示图片"""
cv2.imshow(winname, img)
cv2.waitKey(0)
cv2.destroyAllWindows()
if __name__ == '__main__':
# 加载图片
img = load_image('color_blind_road_2.png')
if img is None:
exit()
# 灰度转换
gray = gray_transform(img)
# 边缘检测
edges = edge_detection(gray)
# 直线检测
img = line_detection(img, edges)
# 阈值分割
thresh = threshold_segmentation(gray)
# 颜色分割
mask = color_segmentation(img, thresh)
# 纹理分割
texture = texture_segmentation(img, thresh)
# 显示结果
show_image(img, 'img')
show_image(thresh, 'thresh')
show_image(mask, 'color')
show_image(texture, 'texture')
```
将下述代码增加显示边缘图像功能: import cv2 import numpy as np from dxfwrite import DXFEngine as dxf img = cv2.imread('ft.jpg', 0) edges = cv2.Canny(img, 100, 200) thresh = cv2.threshold(edges, 27, 255, cv2.THRESH_BINARY)[1] contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) dwg = dxf.drawing('output2.dxf') for contour in contours: if len(contour) > 1: dwg.add(dxf.polyline(contour[:, 0, :])) dwg.save()
可以在代码最后添加以下代码来显示边缘图像:
```
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
完整代码如下:
```
import cv2
import numpy as np
from dxfwrite import DXFEngine as dxf
img = cv2.imread('ft.jpg', 0)
edges = cv2.Canny(img, 100, 200)
thresh = cv2.threshold(edges, 27, 255, cv2.THRESH_BINARY)[1]
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
dwg = dxf.drawing('output2.dxf')
for contour in contours:
if len(contour) > 1:
dwg.add(dxf.polyline(contour[:, 0, :]))
dwg.save()
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
运行代码后,会在生成的 DXF 文件同时显示边缘图像。
相关推荐
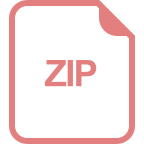
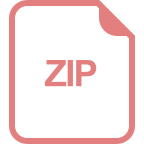
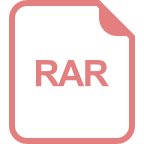













