请还是尝试给我编写一下吧,根据以上信息采用python基于gym构建一个考虑时空信息差异的网约车动态定价和联合调度深度强化学习环境。
时间: 2024-03-06 20:50:51 浏览: 21
好的,我会尝试为您提供一份Python代码的框架,但由于任务比较复杂,这只是一个基本的示例,您需要根据具体的应用场景和算法需求进行修改和优化。代码如下:
```python
import gym
from gym import spaces
import numpy as np
class RideHailingEnv(gym.Env):
def __init__(self):
self.action_space = spaces.MultiDiscrete([15, 15, 15]) # 定义动作空间为15×15×15的矩阵
self.observation_space = spaces.Box(low=0, high=100, shape=(15, 15, 3), dtype=np.float32) # 定义状态空间为15×15×3的矩阵
self.reward_range = (-np.inf, np.inf) # 定义奖励范围为负无穷大到正无穷大
self.state = None # 定义状态为空
self.time_step = 0 # 定义时间步为0
def reset(self):
self.state = np.zeros((15, 15, 3), dtype=np.float32) # 初始化状态为空矩阵
self.time_step = 0 # 时间步归零
return self.state
def step(self, action):
# 根据动作和当前状态更新环境状态
next_state = self.update_state(action)
# 计算奖励
reward = self.calculate_reward(next_state)
# 更新时间步
self.time_step += 1
# 判断是否终止
done = (self.time_step >= 100)
return next_state, reward, done, {}
def update_state(self, action):
# 根据动作和当前状态更新环境状态,具体实现略
return next_state
def calculate_reward(self, next_state):
# 计算奖励,具体实现略
return reward
def render(self, mode='human'):
# 可视化环境状态,具体实现略
return
def close(self):
# 关闭环境
return
class DQNAgent:
def __init__(self, state_size, action_size):
self.state_size = state_size
self.action_size = action_size
# 定义神经网络模型
self.model = self.build_model()
def build_model(self):
# 定义神经网络模型,具体实现略
return model
def act(self, state):
# 根据当前状态选择动作,具体实现略
return action
def train(self, state, action, reward, next_state, done):
# 根据当前状态、动作、奖励、下一个状态和终止标志训练模型,具体实现略
return
# 实例化环境和智能体
env = RideHailingEnv()
agent = DQNAgent(env.observation_space.shape, env.action_space.shape)
# 训练模型
for episode in range(100):
state = env.reset()
for time_step in range(100):
action = agent.act(state)
next_state, reward, done, _ = env.step(action)
agent.train(state, action, reward, next_state, done)
state = next_state
if done:
break
# 测试模型
state = env.reset()
for time_step in range(100):
action = agent.act(state)
next_state, reward, done, _ = env.step(action)
state = next_state
if done:
break
# 关闭环境
env.close()
```
该代码主要定义了一个RideHailingEnv类,该类继承自gym.Env类,实现了reset()、step()、render()和close()等方法,用于重置环境、执行动作、可视化环境状态和关闭环境。该代码还定义了一个DQNAgent类,该类用于实现强化学习算法,包括选择动作、训练模型等方法。在主函数中,该代码首先实例化环境和智能体,然后使用训练数据进行模型训练,最后使用测试数据进行模型测试。
相关推荐
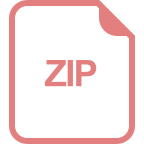
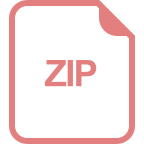














