用c语言编码 用链表实现对学生成绩进行存储、插入及显示功能,学生包括ID和score属性。 结构体类型定义如下: struct grade { int score; char ID[6]; struct grade *next; }; (1)写函数创建单向链表并存储学生成绩信息,输入ID和score按逗号隔开,先输入score后输入ID,当score<0时链表创建完成,并输出创建后的链表信息。 (2)写函数实现在第n个结点后面插入一个新结点,并输出插入新结点后的链表信息。 (3)写函数释放链表所占内存空间。 (在主函数依次调用各函数即可) 输入提示:"输入学生成绩和ID:\n" 输入格式: "%d,%s" 输出提示:"创建的链表:\n" 输出格式:"%d,%s\n" 输入提示:"请输入要插入结点的成绩和ID:\n" 输入格式:"%d,%s" 输入提示:"请输入要插入结点的位置,在这个位置后面插入新结点:\n" 输入格式:%d (提示:插入的位置可能是0,1,...n. 如果要插入位置是其他位置,则输出提示: "插入的节点不存在!") 输出提示:"插入后的链表:\n" 输出格式:"%d,%s\n"
时间: 2023-07-07 08:37:37 浏览: 41
以下是完整的代码,包括三个函数和主函数的调用:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct grade {
int score;
char ID[6];
struct grade *next;
};
struct grade *createList(void);
void insertNode(struct grade *head, int n, int score, char ID[6]);
void freeList(struct grade *head);
int main(void) {
struct grade *head = NULL;
head = createList();
if (head == NULL) {
printf("链表创建失败!\n");
return 1;
}
printf("创建的链表:\n");
struct grade *p = head;
while (p != NULL) {
printf("%d,%s\n", p->score, p->ID);
p = p->next;
}
int n, score;
char ID[6];
printf("请输入要插入结点的成绩和ID:\n");
scanf("%d,%s", &score, ID);
printf("请输入要插入结点的位置,在这个位置后面插入新结点:\n");
scanf("%d", &n);
insertNode(head, n, score, ID);
printf("插入后的链表:\n");
p = head;
while (p != NULL) {
printf("%d,%s\n", p->score, p->ID);
p = p->next;
}
freeList(head);
return 0;
}
struct grade *createList(void) {
struct grade *head = NULL, *p, *tail;
int score;
char ID[6];
printf("输入学生成绩和ID:\n");
scanf("%d,%s", &score, ID);
while (score >= 0) {
p = (struct grade *)malloc(sizeof(struct grade));
if (p == NULL) {
printf("动态内存分配失败!\n");
freeList(head);
return NULL;
}
p->score = score;
strcpy(p->ID, ID);
p->next = NULL;
if (head == NULL) {
head = p;
tail = p;
} else {
tail->next = p;
tail = p;
}
scanf("%d,%s", &score, ID);
}
return head;
}
void insertNode(struct grade *head, int n, int score, char ID[6]) {
struct grade *p = head;
int i = 0;
while (i < n && p != NULL) {
p = p->next;
i++;
}
if (p == NULL) {
printf("插入的节点不存在!\n");
return;
}
struct grade *newNode = (struct grade *)malloc(sizeof(struct grade));
if (newNode == NULL) {
printf("动态内存分配失败!\n");
return;
}
newNode->score = score;
strcpy(newNode->ID, ID);
newNode->next = p->next;
p->next = newNode;
}
void freeList(struct grade *head) {
struct grade *p = head, *q;
while (p != NULL) {
q = p;
p = p->next;
free(q);
}
}
```
函数 `createList` 用于创建链表,并返回链表头指针。输入以逗号隔开的学生ID和成绩,当成绩小于0时结束输入。在输入过程中,动态分配内存并将学生信息存储在链表节点中。如果动态内存分配失败,函数返回 NULL。
函数 `insertNode` 用于在第 n 个节点后面插入一个新节点,新节点的数据为给定的成绩和ID。如果第 n 个节点不存在,函数输出错误信息。在插入过程中,同样动态分配内存。
函数 `freeList` 用于释放链表所占内存空间。从链表头开始遍历,逐个释放节点所占内存。
在主函数中,先调用 `createList` 创建链表并输出链表信息。然后输入要插入的节点的成绩和ID,以及要插入的位置 n,并调用 `insertNode` 进行插入操作。最后调用 `freeList` 释放链表所占内存空间。
需要注意的是,在输入学生ID时,只能输入长度不超过 5 的字符串,否则会发生缓冲区溢出。因此,在定义结构体时,ID 的长度为 6。
相关推荐
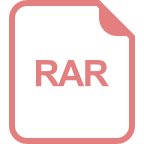
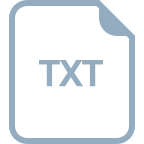
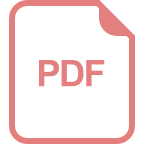














