dfs算法有向图c语言
时间: 2023-07-26 12:11:45 浏览: 48
以下是使用深度优先搜索(DFS)算法遍历有向图的 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 100
typedef struct Node {
int vertex;
struct Node* next;
} Node;
Node* createNode(int v) {
Node* newNode = (Node*) malloc(sizeof(Node));
newNode->vertex = v;
newNode->next = NULL;
return newNode;
}
void addEdge(Node* adj[], int src, int dest) {
Node* newNode = createNode(dest);
newNode->next = adj[src];
adj[src] = newNode;
}
int visited[MAX_VERTICES] = {0};
void DFS(Node* adj[], int start) {
visited[start] = 1;
printf("%d ", start);
Node* temp = adj[start];
while (temp != NULL) {
int connectedVertex = temp->vertex;
if (!visited[connectedVertex]) {
DFS(adj, connectedVertex);
}
temp = temp->next;
}
}
int main() {
Node* adj[MAX_VERTICES];
int numVertices, numEdges;
printf("Enter the number of vertices and edges: ");
scanf("%d%d", &numVertices, &numEdges);
for (int i = 0; i < numVertices; i++) {
adj[i] = NULL;
}
int src, dest;
printf("Enter the edges (source, destination):\n");
for (int i = 0; i < numEdges; i++) {
scanf("%d%d", &src, &dest);
addEdge(adj, src, dest);
}
int startVertex;
printf("Enter the starting vertex for DFS: ");
scanf("%d", &startVertex);
printf("DFS traversal starting from vertex %d: ", startVertex);
DFS(adj, startVertex);
return 0;
}
```
这段代码使用邻接链表来存储有向图,然后使用 DFS 算法遍历图中所有节点。其中,`addEdge` 函数用于向邻接链表中添加边,`DFS` 函数用于递归遍历所有与当前节点相连的未访问过的节点。最后,用户需要输入起始节点,程序将从该节点开始遍历整个图,并输出遍历的结果。
相关推荐
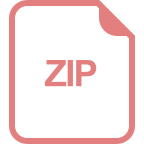
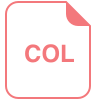
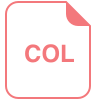
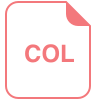
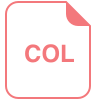










