帮我写一个接收客户端报文并存储成结构体的C语言函数代码
时间: 2023-02-22 21:04:03 浏览: 172
好的,以下是一个简单的例子:
```
#include <stdio.h>
#include <string.h>
struct client_message {
char header[100];
int payload_length;
char payload[1000];
};
int receive_and_store(struct client_message *message) {
// 接收客户端报文并存储到结构体中
int received = 0;
received = recv(client_socket, message, sizeof(struct client_message), 0);
if (received < 0) {
printf("Error receiving message from client\n");
return -1;
}
return 0;
}
```
在上面的代码中,我们定义了一个名为 `client_message` 的结构体,其中包含了报文的头信息(header)、负载长度(payload_length)和负载内容(payload)。然后,我们写了一个名为 `receive_and_store` 的函数,该函数接收一个指向 `client_message` 结构体的指针,并使用 `recv` 函数从客户端接收报文,将其存储到结构体中。如果接收失败,则返回 -1,否则返回 0。
相关问题
帮我写一个接收报文并将报文转为结构体的C语言代码
好的,以下是一个接收报文并将报文转为结构体的简单C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Message {
int id;
char data[256];
};
void receive_message(char *buffer, size_t buffer_size) {
// 这里是模拟接收报文的过程,将报文存储到缓冲区中
// 实际应用中可以根据具体情况自行编写
snprintf(buffer, buffer_size, "%d %s", 123, "hello world");
}
int main() {
char buffer[512];
receive_message(buffer, sizeof(buffer));
struct Message msg;
sscanf(buffer, "%d %s", &msg.id, msg.data);
// 使用 sscanf 函数将报文中的数据解析到结构体中
printf("Received message: id=%d, data=%s\n", msg.id, msg.data);
return 0;
}
```
在这个示例中,我们定义了一个 `struct Message` 结构体来存储报文数据,其中包含一个整型的 `id` 字段和一个长度为 256 的字符数组 `data` 字段。我们还定义了一个 `receive_message` 函数来模拟接收报文的过程,实际应用中可以根据具体情况自行编写。
在 `main` 函数中,我们先定义一个大小为 512 的字符数组 `buffer` 来存储接收到的报文,然后调用 `receive_message` 函数将报文存储到缓冲区中。接着,我们定义一个 `struct Message` 类型的变量 `msg`,使用 `sscanf` 函数将缓冲区中的报文数据解析到结构体中。最后,我们打印出接收到的消息的内容,即 `msg` 结构体中的 `id` 和 `data` 字段。
这只是一个简单的示例代码,实际应用中需要根据具体情况进行调整和优化。
linux C语言利用<time.h>写一个获取时间戳函数,要求此函数能够将当前获取报文的毫秒级时间获取后传给某个结构体里的time成员
可以使用time.h中的time()函数获取当前时间的秒数,再使用gettimeofday()函数获取当前时间的微秒数,最后将其转换为毫秒级时间戳并存储到结构体中的time成员中。
代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/time.h>
#include <time.h>
struct my_struct {
long long time; // 毫秒级时间戳
// 其他成员...
};
void get_timestamp(struct my_struct *s) {
struct timeval tv;
gettimeofday(&tv, NULL);
s->time = ((long long)tv.tv_sec) * 1000 + ((long long)tv.tv_usec) / 1000;
}
int main() {
struct my_struct s;
get_timestamp(&s);
printf("time = %lld\n", s.time);
return 0;
}
```
注意,此方式获取的时间戳是基于系统时间的,如果系统时间被修改,可能会导致该函数获取的时间戳不准确。
阅读全文
相关推荐
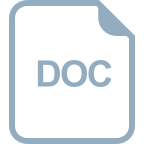
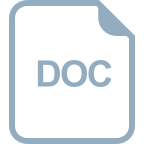
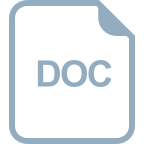

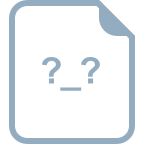
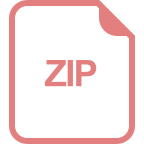
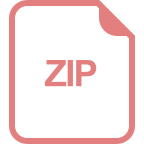









