Cannot find a deserializer for non-concrete Collection type [collection type; class com.google.common.collect.ImmutableList, contains [simple type, class org.eclipse.milo.opcua.sdk.client.api.subscriptions.UaMonitoredItem]]
时间: 2023-12-22 16:06:32 浏览: 296
这个错误通常出现在反序列化一个包含了`ImmutableList`类型的JSON字符串时。`ImmutableList`是Guava库中的一个不可变的列表实现,它是一个抽象类,没有公共的构造函数,因此它不能被直接反序列化。
解决这个问题的方法是,你需要为`ImmutableList`注册一个自定义的反序列化器。你可以通过实现`JsonDeserializer`接口并覆盖`deserialize`方法来创建一个自定义反序列化器。例如:
```java
import java.io.IOException;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.google.common.collect.ImmutableList;
public class ImmutableListDeserializer<T> extends JsonDeserializer<ImmutableList<T>> {
@Override
public ImmutableList<T> deserialize(JsonParser p, DeserializationContext ctxt) throws IOException, JsonProcessingException {
// 从JSON字符串中读取列表元素,并创建一个不可变列表
return ImmutableList.copyOf(p.readValuesAs(getParameterizedType(), new MyListBean()));
}
// 获取列表元素的类型
private Class<T> getParameterizedType() {
// TODO: 这里需要根据具体情况返回列表元素的类型
return null;
}
// 用于读取列表元素的bean类
private static class MyListBean {
// 需要一个空的bean类来读取列表元素
}
}
```
在这个例子中,我们创建了一个`ImmutableListDeserializer`类来反序列化`ImmutableList`类型的对象。在`deserialize`方法中,我们使用`p.readValuesAs`方法从JSON字符串中读取列表元素,并使用`ImmutableList.copyOf`方法创建一个不可变列表。需要注意的是,`ImmutableList`是一个泛型类,因此我们需要在`getParameterizedType`方法中返回列表元素的具体类型。另外,我们还需要一个空的`MyListBean`类来读取列表元素。
完成`ImmutableListDeserializer`类的编写之后,你需要在你的Jackson配置中注册这个反序列化器。例如:
```java
ObjectMapper mapper = new ObjectMapper();
SimpleModule module = new SimpleModule();
module.addDeserializer(ImmutableList.class, new ImmutableListDeserializer<>());
mapper.registerModule(module);
```
在这个例子中,我们创建了一个`ObjectMapper`对象,并注册了一个自定义模块。在这个模块中,我们为`ImmutableList`类型注册了一个自定义反序列化器。最后,我们通过`registerModule`方法将这个模块注册到`ObjectMapper`中。
阅读全文
相关推荐
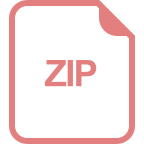
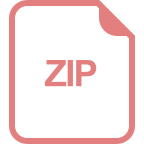
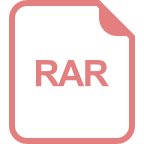
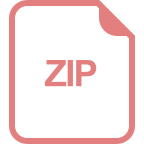
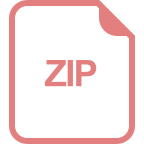
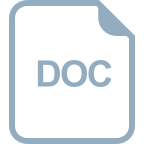
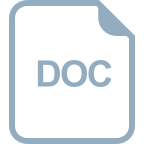
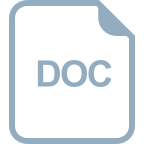








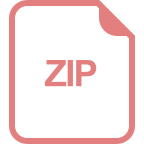