用c sharp实现四叉树的代码
时间: 2023-08-31 09:32:32 浏览: 70
### 回答1:
以下是使用C#实现四叉树的基本代码:
```csharp
public class Quadtree
{
private int MAX_OBJECTS = 10;
private int MAX_LEVELS = 5;
private int level;
private List<GameObject> objects;
private Rect bounds;
private Quadtree[] nodes;
public Quadtree(int level, Rect bounds)
{
this.level = level;
objects = new List<GameObject>();
this.bounds = bounds;
nodes = new Quadtree[4];
}
public void Clear()
{
objects.Clear();
for (int i = 0; i < nodes.Length; i++)
{
if (nodes[i] != null)
{
nodes[i].Clear();
nodes[i] = null;
}
}
}
private void Split()
{
int subWidth = (int)(bounds.width / 2);
int subHeight = (int)(bounds.height / 2);
int x = (int)bounds.x;
int y = (int)bounds.y;
nodes[0] = new Quadtree(level + 1, new Rect(x + subWidth, y, subWidth, subHeight));
nodes[1] = new Quadtree(level + 1, new Rect(x, y, subWidth, subHeight));
nodes[2] = new Quadtree(level + 1, new Rect(x, y + subHeight, subWidth, subHeight));
nodes[3] = new Quadtree(level + 1, new Rect(x + subWidth, y + subHeight, subWidth, subHeight));
}
private int GetIndex(Rect pRect)
{
int index = -1;
double verticalMidpoint = bounds.x + (bounds.width / 2);
double horizontalMidpoint = bounds.y + (bounds.height / 2);
bool topQuadrant = (pRect.y < horizontalMidpoint && pRect.y + pRect.height < horizontalMidpoint);
bool bottomQuadrant = (pRect.y > horizontalMidpoint);
if (pRect.x < verticalMidpoint && pRect.x + pRect.width < verticalMidpoint)
{
if (topQuadrant)
{
index = 1;
}
else if (bottomQuadrant)
{
index = 2;
}
}
else if (pRect.x > verticalMidpoint)
{
if (topQuadrant)
{
index = 0;
}
else if (bottomQuadrant)
{
index = 3;
}
}
return index;
}
public void Insert(GameObject obj)
{
if (nodes[0] != null)
{
int index = GetIndex(obj.GetComponent<RectTransform>().rect);
if (index != -1)
{
nodes[index].Insert(obj);
return;
}
}
objects.Add(obj);
if (objects.Count > MAX_OBJECTS && level < MAX_LEVELS)
{
if (nodes[0] == null)
{
Split();
}
int i = 0;
while (i < objects.Count)
{
int index = GetIndex(objects[i].GetComponent<RectTransform>().rect);
if (index != -1)
{
nodes[index].Insert(objects[i]);
objects.RemoveAt(i);
}
else
{
i++;
}
}
}
}
public List<GameObject> Retrieve(Rect pRect)
{
List<GameObject> returnObjects = new List<GameObject>();
int index = GetIndex(pRect);
### 回答2:
四叉树是一种经典的数据结构,用于处理二维空间的分割和查询。在C#中实现四叉树可以通过定义QuadTree类和QuadTreeNode类来完成。
首先,在QuadTreeNode类中定义四叉树节点的结构。每个节点包含四个子节点、一个父节点和一个矩形边界。矩形边界可以通过左上角和右下角的坐标来表示。
```csharp
class QuadTreeNode
{
// 矩形边界
public double Left;
public double Top;
public double Right;
public double Bottom;
// 子节点
public QuadTreeNode[] Children;
// 父节点
public QuadTreeNode Parent;
public QuadTreeNode(double left, double top, double right, double bottom)
{
Left = left;
Top = top;
Right = right;
Bottom = bottom;
}
}
```
接下来,在QuadTree类中定义四叉树的创建和查询操作。四叉树的创建操作需要递归地将空间划分为四个子节点,并在合适的位置插入数据。查询操作可以根据给定的矩形边界,查找出所有与之相交的节点。
```csharp
class QuadTree
{
private QuadTreeNode root;
public QuadTree(double left, double top, double right, double bottom)
{
root = new QuadTreeNode(left, top, right, bottom);
}
public void Insert(double x, double y, object data)
{
// 在适当的节点插入数据
// ...
}
public List<object> Query(double left, double top, double right, double bottom)
{
List<object> result = new List<object>();
// 根据给定的矩形边界查找所有相交的节点
// ...
return result;
}
}
```
四叉树的具体实现需要根据具体的需求进行调整,包括数据插入的逻辑和查询的实现。上述代码只是一个基本的框架,可以在此基础上进行扩展和优化。
相关推荐
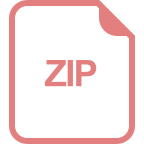
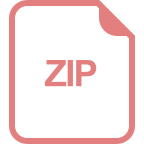
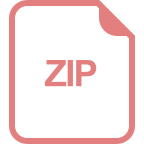














