using System.Collections; using UnityEngine; using UnityEngine.Networking; using UnityEngine.UI; using LitJson; using System.Collections.Generic; public class GetData : MonoBehaviour { public Text resultText; IEnumerator Start() { // 定义接口地址和请求参数 string url = "http://your-interface-url.com"; string json = "{\"lineid\": 27, \"areaid\": 22, \"starttime\": \"2023-05-07 09:54:22\", \"endtime\": \"2023-06-07 09:54:22\", \"datatype\": 1, \"pageSize\": 5, \"pageNumber\": 1 }"; // 构造请求 UnityWebRequest request = new UnityWebRequest(url, "POST"); byte[] bodyRaw = System.Text.Encoding.UTF8.GetBytes(json); request.uploadHandler = (UploadHandler)new UploadHandlerRaw(bodyRaw); request.downloadHandler = (DownloadHandler)new DownloadHandlerBuffer(); request.SetRequestHeader("Content-Type", "application/json"); // 发送请求 yield return request.SendWebRequest(); // 处理返回结果 if (request.result == UnityWebRequest.Result.ConnectionError || request.result == UnityWebRequest.Result.ProtocolError) { Debug.Log(request.error); resultText.text = request.error; } else { // 解析返回的 JSON 数据 string jsonResult = request.downloadHandler.text; JSONObject jsonObject = new JSONObject(jsonResult); // 获取需要的数据,将其显示在文本框中 string text = ""; foreach (JSONObject item in jsonObject["data"]["list"].list) { text += item["doorno"].str + " - " + item["faultname"].str + " - " + item["happentime"].str + "\n"; } resultText.text = text; } } }换掉代码中的result和JSONObject,因为有错误,新代码怎么写
时间: 2024-02-14 13:13:09 浏览: 27
根据您提供的代码,我看到两处可能出现错误的地方:
1. `resultText` 变量可能未被初始化,导致在 `resultText.text` 赋值时出错。
2. `JSONObject` 类型未定义,可能需要使用其他 JSON 解析库。
以下是修改后的代码示例:
```csharp
using System.Collections;
using UnityEngine;
using UnityEngine.Networking;
using UnityEngine.UI;
using LitJson;
using System.Collections.Generic;
public class GetData : MonoBehaviour
{
public Text resultText;
IEnumerator Start()
{
// 定义接口地址和请求参数
string url = "http://your-interface-url.com";
string json = "{\"lineid\": 27, \"areaid\": 22, \"starttime\": \"2023-05-07 09:54:22\", \"endtime\": \"2023-06-07 09:54:22\", \"datatype\": 1, \"pageSize\": 5, \"pageNumber\": 1 }";
// 构造请求
UnityWebRequest request = new UnityWebRequest(url, "POST");
byte[] bodyRaw = System.Text.Encoding.UTF8.GetBytes(json);
request.uploadHandler = (UploadHandler)new UploadHandlerRaw(bodyRaw);
request.downloadHandler = (DownloadHandler)new DownloadHandlerBuffer();
request.SetRequestHeader("Content-Type", "application/json");
// 发送请求
yield return request.SendWebRequest();
// 处理返回结果
if (request.result == UnityWebRequest.Result.ConnectionError || request.result == UnityWebRequest.Result.ProtocolError)
{
Debug.Log(request.error);
resultText.text = request.error;
}
else
{
// 解析返回的 JSON 数据
JsonData jsonData = JsonMapper.ToObject(request.downloadHandler.text);
// 获取需要的数据,将其显示在文本框中
string text = "";
foreach (JsonData item in jsonData["data"]["list"])
{
text += item["doorno"].ToString() + " - " + item["faultname"].ToString() + " - " + item["happentime"].ToString() + "\n";
}
resultText.text = text;
}
}
}
```
注意,这里使用了 LitJson 库来解析 JSON 数据,因此代码中的 `JSONObject` 类型被替换成了 `JsonData` 类型。另外,对于文本框的赋值,我们使用了 `ToString()` 方法而不是 `str` 属性,以确保数据类型正确。
相关推荐
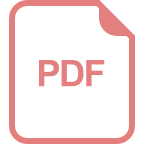













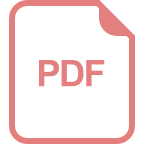
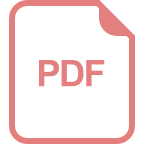
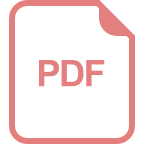