1、设计实现任意两个复数进行4则运算(复数加法、减法、乘法、除法)的Java Web程序。要求采用JavaBean+Jsp+Servlet的MVC设计模式实现。
时间: 2023-12-28 20:04:44 浏览: 52
好的,我会尽力回答你的问题。
首先,我们需要定义一个复数类 Complex,该类包含实部和虚部两个属性,以及复数加减乘除四种运算方法。
```
public class Complex {
private double real;
private double imaginary;
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public Complex add(Complex other) {
double real = this.real + other.real;
double imaginary = this.imaginary + other.imaginary;
return new Complex(real, imaginary);
}
public Complex subtract(Complex other) {
double real = this.real - other.real;
double imaginary = this.imaginary - other.imaginary;
return new Complex(real, imaginary);
}
public Complex multiply(Complex other) {
double real = this.real * other.real - this.imaginary * other.imaginary;
double imaginary = this.real * other.imaginary + this.imaginary * other.real;
return new Complex(real, imaginary);
}
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imaginary * other.imaginary;
double real = (this.real * other.real + this.imaginary * other.imaginary) / denominator;
double imaginary = (this.imaginary * other.real - this.real * other.imaginary) / denominator;
return new Complex(real, imaginary);
}
public double getReal() {
return real;
}
public double getImaginary() {
return imaginary;
}
}
```
然后,我们需要创建一个名为 ComplexCalculator 的 JavaBean,该类包含两个 Complex 属性和四个方法,分别实现复数加减乘除四种运算。
```
public class ComplexCalculator {
private Complex operand1;
private Complex operand2;
public ComplexCalculator() {
operand1 = new Complex(0, 0);
operand2 = new Complex(0, 0);
}
public Complex getOperand1() {
return operand1;
}
public void setOperand1(Complex operand1) {
this.operand1 = operand1;
}
public Complex getOperand2() {
return operand2;
}
public void setOperand2(Complex operand2) {
this.operand2 = operand2;
}
public Complex add() {
return operand1.add(operand2);
}
public Complex subtract() {
return operand1.subtract(operand2);
}
public Complex multiply() {
return operand1.multiply(operand2);
}
public Complex divide() {
return operand1.divide(operand2);
}
}
```
接下来,我们需要创建一个名为 ComplexCalculatorServlet 的 Servlet 类,该类实现了 doGet 方法和 doPost 方法,用于处理 HTTP GET 和 POST 请求。
```
@WebServlet("/complexcalculator")
public class ComplexCalculatorServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ComplexCalculator calculator = new ComplexCalculator();
request.setAttribute("calculator", calculator);
request.getRequestDispatcher("/complexcalculator.jsp").forward(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
ComplexCalculator calculator = new ComplexCalculator();
calculator.setOperand1(new Complex(Double.parseDouble(request.getParameter("real1")),
Double.parseDouble(request.getParameter("imaginary1"))));
calculator.setOperand2(new Complex(Double.parseDouble(request.getParameter("real2")),
Double.parseDouble(request.getParameter("imaginary2"))));
String operation = request.getParameter("operation");
Complex result = null;
switch (operation) {
case "add":
result = calculator.add();
break;
case "subtract":
result = calculator.subtract();
break;
case "multiply":
result = calculator.multiply();
break;
case "divide":
result = calculator.divide();
break;
}
request.setAttribute("calculator", calculator);
request.setAttribute("result", result);
request.getRequestDispatcher("/complexcalculator.jsp").forward(request, response);
}
}
```
最后,我们需要创建一个名为 complexcalculator.jsp 的 JSP 页面,该页面包含一个表单,用于输入两个复数和选择运算符,以及一个用于显示结果的元素。
```
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Complex Calculator</title>
</head>
<body>
<h1>Complex Calculator</h1>
<form method="post" action="complexcalculator">
<label>
Operand 1:
<input type="text" name="real1" value="${calculator.operand1.real}"/> +
<input type="text" name="imaginary1" value="${calculator.operand1.imaginary}"/>i
</label>
<br/>
<label>
Operand 2:
<input type="text" name="real2" value="${calculator.operand2.real}"/> +
<input type="text" name="imaginary2" value="${calculator.operand2.imaginary}"/>i
</label>
<br/>
<label>
Operation:
<select name="operation">
<option value="add">+</option>
<option value="subtract">-</option>
<option value="multiply">*</option>
<option value="divide">/</option>
</select>
</label>
<br/>
<input type="submit" value="Calculate"/>
</form>
<%
Complex result = (Complex) request.getAttribute("result");
if (result != null) {
%>
<p>Result: <%= result.getReal() %> + <%= result.getImaginary() %>i</p>
<%
}
%>
</body>
</html>
```
以上就是实现任意两个复数进行4则运算的 Java Web 程序的全部代码,该程序采用了 JavaBean+Jsp+Servlet 的 MVC 设计模式实现,可以通过部署到 Tomcat 等 Web 服务器上进行访问和使用。
相关推荐
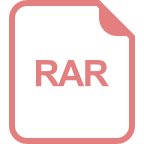














