stm32cubemx通过PWM控制42步进电机寸动的中断回调函数怎么写
时间: 2023-11-18 13:49:14 浏览: 114
下面是一个简单的示例代码,用于在stm32cubemx上使用PWM控制42步进电机:
首先,需要在stm32cubemx中配置PWM和中断。在main.c文件中,需要添加以下代码:
```c
#include "main.h"
#include "stm32f4xx_hal.h"
/* TIM handler declaration */
TIM_HandleTypeDef htim1;
/* PWM Configuration */
TIM_OC_InitTypeDef sConfigOC;
/* Step counter */
uint8_t step_counter = 0;
/* Step sequence */
uint8_t step_sequence[] = {0x01, 0x03, 0x02, 0x06, 0x04, 0x0C, 0x08, 0x09};
/* Function prototypes */
void HAL_TIM_PWM_MspInit(TIM_HandleTypeDef *htim);
void HAL_TIM_PWM_MspDeInit(TIM_HandleTypeDef *htim);
void HAL_TIM_PWM_PulseFinishedCallback(TIM_HandleTypeDef *htim);
/* PWM callback function */
void HAL_TIM_PWM_PulseFinishedCallback(TIM_HandleTypeDef *htim)
{
/* Disable PWM output */
HAL_TIM_PWM_Stop(&htim1, TIM_CHANNEL_1);
/* Increment step counter */
step_counter++;
/* Check if step counter is greater than step sequence length */
if (step_counter >= sizeof(step_sequence))
{
/* Reset step counter */
step_counter = 0;
}
/* Set new PWM duty cycle based on step sequence */
sConfigOC.Pulse = step_sequence[step_counter];
/* Update PWM duty cycle */
HAL_TIM_PWM_ConfigChannel(&htim1, &sConfigOC, TIM_CHANNEL_1);
/* Enable PWM output */
HAL_TIM_PWM_Start(&htim1, TIM_CHANNEL_1);
}
/* PWM initialization function */
static void MX_TIM1_Init(void)
{
/* Timer handler declaration */
TIM_ClockConfigTypeDef sClockSourceConfig = {0};
TIM_MasterConfigTypeDef sMasterConfig = {0};
/* Enable TIM1 clock */
__HAL_RCC_TIM1_CLK_ENABLE();
/* Initialize TIM1 handler */
htim1.Instance = TIM1;
htim1.Init.Prescaler = 0;
htim1.Init.CounterMode = TIM_COUNTERMODE_UP;
htim1.Init.Period = 255;
htim1.Init.ClockDivision = TIM_CLOCKDIVISION_DIV1;
htim1.Init.RepetitionCounter = 0;
htim1.Init.AutoReloadPreload = TIM_AUTORELOAD_PRELOAD_DISABLE;
/* Initialize PWM configuration */
sConfigOC.OCMode = TIM_OCMODE_PWM1;
sConfigOC.Pulse = 0;
sConfigOC.OCPolarity = TIM_OCPOLARITY_HIGH;
sConfigOC.OCFastMode = TIM_OCFAST_DISABLE;
/* Initialize TIM1 PWM */
HAL_TIM_PWM_Init(&htim1);
/* Configure TIM1 PWM channel */
HAL_TIM_PWM_ConfigChannel(&htim1, &sConfigOC, TIM_CHANNEL_1);
/* Configure TIM1 master mode */
sMasterConfig.MasterOutputTrigger = TIM_TRGO_OC1REF;
sMasterConfig.MasterSlaveMode = TIM_MASTERSLAVEMODE_DISABLE;
HAL_TIMEx_MasterConfigSynchronization(&htim1, &sMasterConfig);
/* Configure TIM1 clock source */
sClockSourceConfig.ClockSource = TIM_CLOCKSOURCE_INTERNAL;
HAL_TIM_ConfigClockSource(&htim1, &sClockSourceConfig);
/* Enable PWM output */
HAL_TIM_PWM_Start(&htim1, TIM_CHANNEL_1);
/* Register PWM callback function */
HAL_TIM_PWM_RegisterCallback(&htim1, HAL_TIM_PWM_PULSEFINISHED_CB_ID, HAL_TIM_PWM_PulseFinishedCallback);
/* Enable PWM interrupt */
HAL_TIM_PWM_Start_IT(&htim1, TIM_CHANNEL_1);
}
/* PWM MSP initialization function */
void HAL_TIM_PWM_MspInit(TIM_HandleTypeDef *htim)
{
/* TIM1 clock enable */
__HAL_RCC_TIM1_CLK_ENABLE();
/* TIM1 GPIO Configuration */
GPIO_InitTypeDef GPIO_InitStruct = {0};
__HAL_RCC_GPIOA_CLK_ENABLE();
GPIO_InitStruct.Pin = GPIO_PIN_8;
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
GPIO_InitStruct.Alternate = GPIO_AF1_TIM1;
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
}
/* PWM MSP de-initialization function */
void HAL_TIM_PWM_MspDeInit(TIM_HandleTypeDef *htim)
{
/* TIM1 GPIO de-configuration */
HAL_GPIO_DeInit(GPIOA, GPIO_PIN_8);
/* TIM1 clock disable */
__HAL_RCC_TIM1_CLK_DISABLE();
}
/* System initialization function */
void System_Init(void)
{
/* Reset all peripherals, initialize the Flash interface and the Systick */
HAL_Init();
/* Initialize PWM */
MX_TIM1_Init();
/* Start PWM output */
HAL_TIM_PWM_Start(&htim1, TIM_CHANNEL_1);
}
int main(void)
{
/* System initialization */
System_Init();
/* Infinite loop */
while (1)
{
/* Do nothing */
}
}
```
在这个示例中,我们使用了STM32F4系列的TIM1作为PWM定时器,使用了PA8引脚作为PWM输出。步进电机的步序列存储在step_sequence数组中,步进电机的步数存储在step_counter变量中。
在HAL_TIM_PWM_PulseFinishedCallback回调函数中,我们首先停止PWM输出,然后根据步序列设置新的PWM占空比,并重新启动PWM输出。在这个过程中,我们还递增了step_counter变量,并检查它是否超过了步序列的长度。如果超过了步序列的长度,我们就将step_counter重置为0。
在MX_TIM1_Init函数中,我们初始化了PWM定时器和PWM通道,并配置了PWM的占空比和时钟源。我们还使用HAL_TIM_PWM_RegisterCallback函数注册了PWM回调函数,并使用HAL_TIM_PWM_Start_IT函数启用了PWM中断。
最后,在System_Init函数中,我们初始化了系统和PWM,并在主循环中不做任何事情。
相关推荐
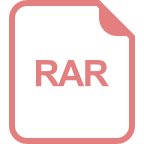
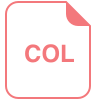
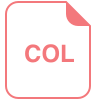
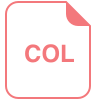
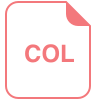
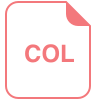









