写一段两层卷积代码,使用pytorch
时间: 2023-10-24 09:05:41 浏览: 46
以下是一段使用PyTorch实现的两层卷积代码示例:
```python
import torch
import torch.nn as nn
class ConvNet(nn.Module):
def __init__(self, num_classes=10):
super(ConvNet, self).__init__()
self.layer1 = nn.Sequential(
nn.Conv2d(in_channels=3, out_channels=32, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(32),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2))
self.layer2 = nn.Sequential(
nn.Conv2d(in_channels=32, out_channels=64, kernel_size=3, stride=1, padding=1),
nn.BatchNorm2d(64),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2, stride=2))
self.fc = nn.Linear(in_features=64*8*8, out_features=num_classes)
def forward(self, x):
out = self.layer1(x)
out = self.layer2(out)
out = out.reshape(out.size(0), -1)
out = self.fc(out)
return out
```
在这个示例中,我们定义了一个ConvNet类,该类包含两个卷积层和一个全连接层。第一层卷积层由一个3x3的卷积核组成,输出通道数为32,带有一个batch normalization和ReLU激活函数,最后通过一个2x2的最大池化层进行下采样。第二层卷积层与第一层卷积层类似,但是输出通道数为64。最后,我们将输出压缩成一个向量,并通过全连接层将其映射到类别数(在此示例中为10)。
在forward方法中,我们首先通过第一层和第二层卷积层传递输入张量x,并使用reshape方法将输出张量展平。最后,我们将其传递到全连接层中,以获得最终的类别预测。
相关推荐
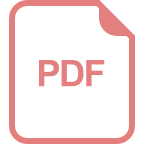
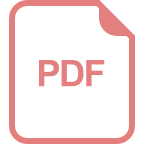
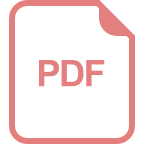














