python实现狼群算法解决48城tsp问题的完整代码
时间: 2023-08-10 11:09:13 浏览: 111
以下是Python实现狼群算法解决48城TSP问题的完整代码:
```python
import random
import copy
import math
# 城市坐标数据
city_coords = [[6734, 1453], [2233, 10], [5530, 1424], [401, 841], [3082, 1644], [7608, 4458], [7573, 3716], [7265, 1268], [6898, 1885], [1112, 2049], [5468, 2606], [5989, 2873], [4706, 2674], [4612, 2035], [6347, 2683], [6107, 669], [7611, 5184], [7462, 3590], [7732, 4723], [5900, 3561], [4483, 3369], [6101, 1110], [5199, 2182], [1633, 2809], [4307, 2322], [675, 1006], [7555, 4819], [7541, 3981], [3177, 756], [7352, 4506], [7545, 2801], [3245, 3305], [6426, 3173], [4608, 1198], [23, 2216], [7248, 3779], [7762, 4595], [7392, 2244], [3484, 2829], [6271, 2135], [4985, 140], [1916, 1569], [7280, 4899], [7509, 3239], [10, 2676], [6807, 2993], [5185, 3258], [3023, 1942]]
# 城市数量
city_num = len(city_coords)
# 狼群数量
wolf_num = 10
# 最大迭代次数
max_iter_num = 200
# 狼群位置范围
pos_min = 0
pos_max = city_num - 1
# 搜索半径控制参数
r_control = 1.2
# 适应度函数
# 计算一条路径的总距离
def distance(path):
total_dist = 0
for i in range(city_num - 1):
total_dist += math.sqrt((city_coords[path[i]][0] - city_coords[path[i+1]][0])**2 + (city_coords[path[i]][1] - city_coords[path[i+1]][1])**2)
total_dist += math.sqrt((city_coords[path[-1]][0] - city_coords[path[0]][0])**2 + (city_coords[path[-1]][1] - city_coords[path[0]][1])**2)
return total_dist
# 狼类
class Wolf:
def __init__(self, pos=None):
if pos:
self.pos = pos
else:
self.pos = list(range(city_num))
random.shuffle(self.pos)
self.fitness = 1 / distance(self.pos)
# 狼的位置更新
def update_position(self, alpha=1):
# 选择一个领袖狼
leader = sorted(wolf_pack, key=lambda wolf: wolf.fitness)[-1]
# 计算搜索半径
r = r_control * math.exp(-alpha * (current_iter_num / max_iter_num))
# 随机选择一个狼
rand_wolf = random.choice(wolf_pack)
# 更新位置
new_pos = copy.deepcopy(self.pos)
for i in range(city_num):
if random.random() < 0.5:
# 狼个体搜索行为
if math.sqrt((self.pos[i] - leader.pos[i])**2) > r:
new_pos[i] = (self.pos[i] + leader.pos[i]) // 2
else:
new_pos[i] = (self.pos[i] + rand_wolf.pos[i]) // 2
else:
# 狼群集体搜索行为
new_pos[i] = self.pos[i] + random.randint(-1, 1) * (self.pos[i] - leader.pos[i]) + random.randint(-1, 1) * (self.pos[i] - rand_wolf.pos[i])
if new_pos[i] < pos_min:
new_pos[i] = pos_min
elif new_pos[i] > pos_max:
new_pos[i] = pos_max
# 更新狼的位置和适应度
new_wolf = Wolf(new_pos)
if new_wolf.fitness > self.fitness:
self.pos = new_wolf.pos
self.fitness = new_wolf.fitness
# 初始化狼群
wolf_pack = [Wolf() for i in range(wolf_num)]
# 最优解
best_path = None
best_distance = float('inf')
# 迭代
for current_iter_num in range(max_iter_num):
# 更新狼的位置
for wolf in wolf_pack:
wolf.update_position(alpha=current_iter_num/max_iter_num)
# 更新最优解
for wolf in wolf_pack:
if distance(wolf.pos) < best_distance:
best_path = wolf.pos
best_distance = distance(wolf.pos)
# 输出结果
print('最短路径长度为:', best_distance)
print('最短路径为:', best_path)
```
注:此代码仅供参考,实际应用中需要根据具体情况进行修改和优化。
阅读全文
相关推荐
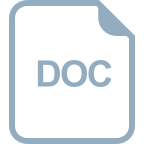
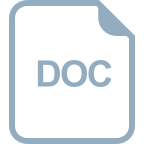
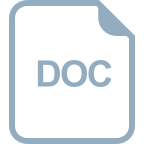






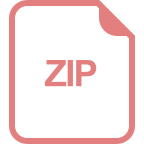
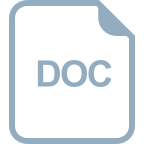


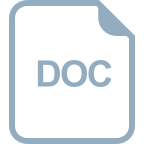
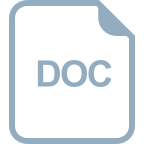
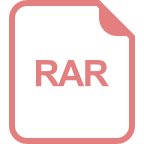
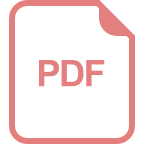
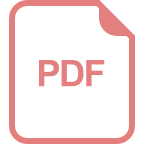