class ImageDataset(Dataset): def __init__(self, image_paths): super().__init__() self.image_paths = image_paths self.transform = albumentations.Compose([ albumentations.RandomCrop(height=128, width=128) ]) self.cutout = albumentations.Cutout(num_holes=12, max_h_size=24, max_w_size=24, p=1.0, fill_value=1.0) def __len__(self): return len(self.image_paths) def __getitem__(self, index): image = cv2.imread(self.image_paths[index]) image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) / 255.0 image = self.transform(image=image)['image'] image_cutout = self.cutout(image=image)['image'] image = image.reshape((1, image.shape[0], image.shape[1])) image_cutout = image_cutout.reshape((1, image_cutout.shape[0], image_cutout.shape[1])) mask = (image_cutout != 1.0) * 1.0 return image, image_cutout, mask
时间: 2024-02-15 11:26:27 浏览: 44
这段代码是一个PyTorch中的自定义数据集类(ImageDataset),用于加载图像数据并进行数据增强操作。以下是对每个方法的解释:
1. `__init__(self, image_paths)`:构造函数,接收一个包含所有图像路径的列表作为输入参数,并将其存储在成员变量`self.image_paths`中。
2. `__len__(self)`:返回数据集中图像的数量。
3. `__getitem__(self, index)`:根据给定的索引,加载对应路径的图像,并进行数据增强操作。具体步骤如下:
- 使用OpenCV的`cv2.imread()`函数加载图像。
- 将图像从BGR格式转换为灰度图像,并将像素值归一化到0到1之间。
- 使用albumentations库中的`Compose()`函数创建一个数据增强管道,其中包含一个随机裁剪操作(RandomCrop)。
- 使用`Cutout()`函数在图像中随机遮挡12个小区域,模拟数据缺失的情况,从而增强模型的泛化性能。
- 将图像和遮挡后的图像reshape为单通道的张量,并创建一个二值掩码(mask)表示遮挡的区域。
- 返回原图、遮挡后的图像和二值掩码。
相关问题
class ImageDataset(Dataset): def init( self, resolution, image_paths, classes=None, shard=0, num_shards=1, random_crop=False, random_flip=True, ): super().init() self.resolution = resolution self.local_images = image_paths[shard:][::num_shards] self.local_classes = None if classes is None else classes[shard:][::num_shards] self.random_crop = random_crop self.random_flip = random_flip 里面的self.resolution如何实例化
`self.resolution` 是在 `ImageDataset` 类的构造函数中传递的参数,因此需要在创建 `ImageDataset` 对象时进行实例化。例如:
```
dataset = ImageDataset(resolution=(256, 256), image_paths=image_paths, classes=classes, shard=0, num_shards=1, random_crop=True, random_flip=True)
```
在这个例子中,`resolution` 被实例化为一个元组 `(256, 256)`,并传递给了 `ImageDataset` 的构造函数。
tiny imagenet 训练_Dataloader读取图片集并训练网络python实现
以下是使用PyTorch实现Dataloader读取Tiny ImageNet数据集并训练网络的示例代码:
```python
import torch
import torchvision
import torchvision.transforms as transforms
from torch.utils.data import Dataset, DataLoader
from torch.utils.data.sampler import SubsetRandomSampler
# 定义自定义数据集类
class TinyImageNetDataset(Dataset):
def __init__(self, data_dir, transform=None):
self.data_dir = data_dir
self.transform = transform
self.image_paths = []
self.labels = []
with open(data_dir + '/wnids.txt', 'r') as f:
self.classes = [line.strip() for line in f.readlines()]
for i, cls in enumerate(self.classes):
for img_file in os.listdir(data_dir + '/train/' + cls + '/images/'):
self.image_paths.append(data_dir + '/train/' + cls + '/images/' + img_file)
self.labels.append(i)
def __len__(self):
return len(self.labels)
def __getitem__(self, idx):
image_path = self.image_paths[idx]
image = Image.open(image_path).convert('RGB')
label = self.labels[idx]
if self.transform:
image = self.transform(image)
return image, label
# 定义数据增强和预处理操作
transform_train = transforms.Compose([
transforms.RandomCrop(64, padding=4),
transforms.RandomHorizontalFlip(),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
transform_test = transforms.Compose([
transforms.Resize(64),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
# 创建训练、验证和测试数据集的实例
train_dataset = TinyImageNetDataset(data_dir='/path/to/tiny-imagenet-200', transform=transform_train)
val_dataset = TinyImageNetDataset(data_dir='/path/to/tiny-imagenet-200', transform=transform_test)
test_dataset = TinyImageNetDataset(data_dir='/path/to/tiny-imagenet-200', transform=transform_test)
# 创建随机子采样器
train_sampler = SubsetRandomSampler(range(100000))
val_sampler = SubsetRandomSampler(range(10000))
test_sampler = SubsetRandomSampler(range(10000))
# 创建Dataloader
train_loader = DataLoader(train_dataset, batch_size=128, sampler=train_sampler, num_workers=4)
val_loader = DataLoader(val_dataset, batch_size=128, sampler=val_sampler, num_workers=4)
test_loader = DataLoader(test_dataset, batch_size=128, sampler=test_sampler, num_workers=4)
# 定义神经网络模型
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(128, 256, kernel_size=3, stride=1, padding=1)
self.fc1 = nn.Linear(256 * 8 * 8, 1024)
self.fc2 = nn.Linear(1024, 200)
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.max_pool2d(x, kernel_size=2, stride=2)
x = F.relu(self.conv2(x))
x = F.max_pool2d(x, kernel_size=2, stride=2)
x = F.relu(self.conv3(x))
x = F.max_pool2d(x, kernel_size=2, stride=2)
x = x.view(-1, 256 * 8 * 8)
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
# 实例化神经网络模型、损失函数和优化器
net = Net()
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(net.parameters(), lr=0.001, momentum=0.9)
# 训练循环
for epoch in range(10):
running_loss = 0.0
for i, data in enumerate(train_loader, 0):
inputs, labels = data
optimizer.zero_grad()
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
if i % 100 == 99:
print('[%d, %5d] loss: %.3f' % (epoch + 1, i + 1, running_loss / 100))
running_loss = 0.0
# 验证和测试循环
correct = 0
total = 0
with torch.no_grad():
for data in val_loader:
images, labels = data
outputs = net(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
val_accuracy = correct / total
print('Validation accuracy: %.2f %%' % (100 * val_accuracy))
correct = 0
total = 0
with torch.no_grad():
for data in test_loader:
images, labels = data
outputs = net(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
test_accuracy = correct / total
print('Test accuracy: %.2f %%' % (100 * test_accuracy))
```
注意:上述代码仅供参考,实际使用时需要根据自己的需求进行修改。
相关推荐
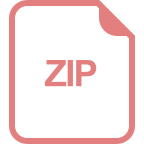











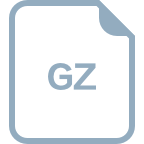