用C语言编写一个程序实现两个一元多项式相乘。 【输入形式】 首先输入第一个多项式中系数不为0的项的个数,然后在新的一行上输入以空格分隔的整数,代表一个多项式,且该多项式中各项的系数均为0或正整数,最高幂次不超过50。对于多项式 anxn + an-1xn-1+ ... + a1x1 + a0x0 (n<50)的输入方法如下: an n an-1 n-1 ... a1 1 a0 0 即相邻两个整数分别表示表达式中一项的系数和指数。在输入中只出现系数不为0的项。 按照上述方式再输入第二个多项式。 【输出形式】 将运算结果输出到屏幕。将系数不为0的项按指数从高到低的顺序输出,每次输出其系数和指数,均以一个空格分隔。最后要求换行。
时间: 2023-12-03 22:42:47 浏览: 217
以下是实现两个一元多项式相乘的C语言程序:
```c
#include <stdio.h>
#define MAX_DEGREE 50
typedef struct {
int coef[MAX_DEGREE+1]; // 系数数组,下标表示指数
int degree; // 最高项的指数
} Poly;
void init_poly(Poly *p) {
int i;
for (i = 0; i <= MAX_DEGREE; i++) {
p->coef[i] = 0;
}
p->degree = 0;
}
void read_poly(Poly *p) {
int n, coef, exp;
scanf("%d", &n);
init_poly(p);
while (n--) {
scanf("%d%d", &coef, &exp);
p->coef[exp] = coef;
if (exp > p->degree) {
p->degree = exp;
}
}
}
void print_poly(Poly *p) {
int i, printed = 0;
for (i = p->degree; i >= 0; i--) {
if (p->coef[i] != 0) {
printf("%d %d ", p->coef[i], i);
printed = 1;
}
}
if (!printed) {
printf("0 0");
}
printf("\n");
}
void add_poly(Poly *a, Poly *b, Poly *c) {
int i;
init_poly(c);
c->degree = a->degree > b->degree ? a->degree : b->degree;
for (i = c->degree; i >= 0; i--) {
c->coef[i] = a->coef[i] + b->coef[i];
}
}
void multiply_poly(Poly *a, Poly *b, Poly *c) {
int i, j;
init_poly(c);
c->degree = a->degree + b->degree;
for (i = 0; i <= a->degree; i++) {
for (j = 0; j <= b->degree; j++) {
c->coef[i+j] += a->coef[i] * b->coef[j];
}
}
}
int main() {
Poly a, b, c;
read_poly(&a);
read_poly(&b);
multiply_poly(&a, &b, &c);
print_poly(&c);
return 0;
}
```
程序中定义了一个多项式的结构体类型Poly,包含一个系数数组和最高项的指数。init_poly函数用于初始化一个多项式,read_poly函数用于读入一个多项式的系数和指数,print_poly函数用于打印一个多项式,add_poly函数用于计算两个多项式的和,multiply_poly函数用于计算两个多项式的积。
在main函数中,我们先读入两个多项式,然后调用multiply_poly函数计算它们的积,最后打印结果。
阅读全文
相关推荐
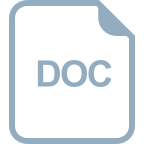
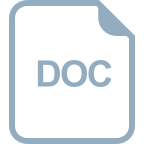
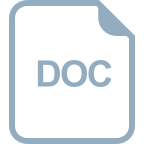
















