int button_state = GPIO_PIN_RESET; int button_prev_state = GPIO_PIN_RESET; int led_state = GPIO_PIN_RESET; while (1) { button_state = HAL_GPIO_ReadPin(GPIOC, GPIO_PIN_13); if (button_state != button_prev_state) { button_prev_state = button_state; if (button_state == GPIO_PIN_SET) { led_state = !led_state; if (led_state == GPIO_PIN_SET) { HAL_GPIO_WritePin(GPIOA, GPIO_PIN_5, GPIO_PIN_SET); } else { HAL_GPIO_WritePin(GPIOA, GPIO_PIN_5, GPIO_PIN_RESET); } } HAL_Delay(200); } }
时间: 2024-04-28 07:19:16 浏览: 88
这段代码是一个基础的嵌入式系统的循环程序,主要实现了一个按键控制LED灯的开关。代码中使用了 STM32 HAL 库,通过读取 GPIOC 的 13 号引脚的状态来检测按键是否被按下,当检测到按键状态发生变化时,根据当前 LED 灯的状态来切换灯的开关状态,并将开关状态写入 GPIOA 的 5 号引脚。这个循环程序会一直运行,每 200ms 检测一次按键状态。
相关问题
void button_handler(struct Button* handle) { uint8_t read_gpio_level = handle->hal_button_Level(handle->button_id); //ticks counter working.. if((handle->state) > 0) handle->ticks++; /*------------button debounce handle---------------*/ if(read_gpio_level != handle->button_level) { //not equal to prev one //continue read 3 times same new level change if(++(handle->debounce_cnt) >= DEBOUNCE_TICKS) { handle->button_level = read_gpio_level; handle->debounce_cnt = 0; } } else { //leved not change ,counter reset. handle->debounce_cnt = 0; } /*-----------------State machine-------------------*/ switch (handle->state) { case 0: if(handle->button_level == handle->active_level) { //start press down handle->event = (uint8_t)PRESS_DOWN; EVENT_CB(PRESS_DOWN); handle->ticks = 0; handle->repeat = 1; handle->state = 1; } else { handle->event = (uint8_t)NONE_PRESS; } break; case 1: if(handle->button_level != handle->active_level) { //released press up handle->event = (uint8_t)PRESS_UP; EVENT_CB(PRESS_UP); handle->ticks = 0; handle->state = 2; } else if(handle->ticks > LONG_TICKS) { handle->event = (uint8_t)LONG_PRESS_START; EVENT_CB(LONG_PRESS_START); handle->state = 5; } break; case 2: if(handle->button_level == handle->active_level) { //press down again handle->event = (uint8_t)PRESS_DOWN; EVENT_CB(PRESS_DOWN); handle->repeat++; EVENT_CB(PRESS_REPEAT); // repeat hit handle->ticks = 0; handle->state = 3; } else if(handle->ticks > SHORT_TICKS) { //released timeout if(handle->repeat == 1) { handle->event = (uint8_t)SINGLE_CLICK; EVENT_CB(SINGLE_CLICK); } else if(handle->repeat == 2) { handle->event = (uint8_t)DOUBLe_CLICK; EVENT_CB(DOUBLe_CLICK); // repeat hit } handle->state = 0; } break; case 3: if(handle->button_level != handle->active_level) { //released press up handle->event = (uint8_t)PRESS_UP; EVENT_CB(PRESS_UP); if(handle->ticks < SHORT_TICKS) { handle->ticks = 0; handle->state = 2; //repeat press } else { handle->state = 0; } }else if(handle->ticks > SHORT_TICKS){ // long press up handle->state = 0; } break; case 5: if(handle->button_level == handle->active_level) { //continue hold trigger handle->event = (uint8_t)LONG_PRESS_HOLD; EVENT_CB(LONG_PRESS_HOLD); } else { //releasd handle->event = (uint8_t)PRESS_UP; EVENT_CB(PRESS_UP); handle->state = 0; //reset } break; default: handle->state = 0; //reset break; } }帮我详细注释这段代码
这段代码是一个按键处理函数,主要用于处理按键的状态和事件。下面是对代码的详细注释:
```c
void button_handler(struct Button* handle) {
// 读取按键GPIO端口的电平值
uint8_t read_gpio_level = handle->hal_button_Level(handle->button_id);
// 计数器开始工作
if((handle->state) > 0) handle->ticks++;
/*------------按键消抖处理---------------*/
if(read_gpio_level != handle->button_level) { //如果读到的电平值不同于之前的
//连续读取3次相同的电平值
if(++(handle->debounce_cnt) >= DEBOUNCE_TICKS) {
handle->button_level = read_gpio_level; //更新电平值
handle->debounce_cnt = 0; //重置消抖计数器
}
} else { //如果电平值没有变化,重置消抖计数器
handle->debounce_cnt = 0;
}
/*-----------------状态机处理-------------------*/
switch (handle->state) {
case 0: //初始状态
if(handle->button_level == handle->active_level) { //按键按下
handle->event = (uint8_t)PRESS_DOWN; //设置按键事件
EVENT_CB(PRESS_DOWN); //触发事件回调函数
handle->ticks = 0; //重置计数器
handle->repeat = 1; //重置按键重复次数
handle->state = 1; //进入下一个状态
} else {
handle->event = (uint8_t)NONE_PRESS; //没有按键按下
}
break;
case 1: //按键按下状态
if(handle->button_level != handle->active_level) { //按键松开
handle->event = (uint8_t)PRESS_UP; //设置按键事件
EVENT_CB(PRESS_UP); //触发事件回调函数
handle->ticks = 0; //重置计数器
handle->state = 2; //进入下一个状态
} else if(handle->ticks > LONG_TICKS) { //按键按下超过长按时间
handle->event = (uint8_t)LONG_PRESS_START; //设置按键事件
EVENT_CB(LONG_PRESS_START); //触发事件回调函数
handle->state = 5; //进入下一个状态
}
break;
case 2: //单次按键按下后的状态
if(handle->button_level == handle->active_level) { //按键再次按下
handle->event = (uint8_t)PRESS_DOWN; //设置按键事件
EVENT_CB(PRESS_DOWN); //触发事件回调函数
handle->repeat++; //增加按键重复次数
EVENT_CB(PRESS_REPEAT); //触发按键重复事件回调函数
handle->ticks = 0; //重置计数器
handle->state = 3; //进入下一个状态
} else if(handle->ticks > SHORT_TICKS) { //按键松开超过短按时间
if(handle->repeat == 1) { //单击事件
handle->event = (uint8_t)SINGLE_CLICK; //设置按键事件
EVENT_CB(SINGLE_CLICK); //触发事件回调函数
} else if(handle->repeat == 2) { //双击事件
handle->event = (uint8_t)DOUBLE_CLICK; //设置按键事件
EVENT_CB(DOUBLE_CLICK); //触发事件回调函数
}
handle->state = 0; //回到初始状态
}
break;
case 3: //按键重复状态
if(handle->button_level != handle->active_level) { //按键松开
handle->event = (uint8_t)PRESS_UP; //设置按键事件
EVENT_CB(PRESS_UP); //触发事件回调函数
if(handle->ticks < SHORT_TICKS) { //按键松开时间小于短按时间
handle->ticks = 0; //重置计数器
handle->state = 2; //回到单次按键按下后的状态
} else { //按键松开时间大于等于短按时间
handle->state = 0; //回到初始状态
}
} else if(handle->ticks > SHORT_TICKS){ //按键按下时间大于短按时间
handle->state = 0; //回到初始状态
}
break;
case 5: //长按状态
if(handle->button_level == handle->active_level) { //按键继续按下
handle->event = (uint8_t)LONG_PRESS_HOLD; //设置按键事件
EVENT_CB(LONG_PRESS_HOLD); //触发事件回调函数
} else { //按键松开
handle->event = (uint8_t)PRESS_UP; //设置按键事件
EVENT_CB(PRESS_UP); //触发事件回调函数
handle->state = 0; //回到初始状态
}
break;
default: //其他状态,回到初始状态
handle->state = 0;
break;
}
}
```
int main(void) { int16_t ax, ay, az; // 初始化MPU6050 MPU6050_Init(); while (1) { // 读取加速度数据 MPU6050_Read_Accel(&ax, &ay, &az); // 处理数据 // ... HAL_Delay(10); } }对ax,ay进行高通滤波后对串口输出
您可以使用以下代码实现:
```c
#include "stdio.h"
#include "stdlib.h"
#include "stdint.h"
#include "stm32f1xx_hal.h"
#define ACCEL_THRESHOLD 100
I2C_HandleTypeDef hi2c1;
UART_HandleTypeDef huart1;
void SystemClock_Config(void);
static void MX_GPIO_Init(void);
static void MX_USART1_UART_Init(void);
static void MX_I2C1_Init(void);
int16_t ax, ay, az;
int16_t ax_hpf, ay_hpf;
void MPU6050_Init(void) {
// 初始化MPU6050的I2C通信
// ...
}
void MPU6050_Read_Accel(int16_t *ax, int16_t *ay, int16_t *az) {
// 读取加速度数据
// ...
}
int16_t HighPassFilter(int16_t input, int16_t prev_output) {
int16_t output = (2 * prev_output - input) / 2;
return output;
}
int main(void) {
HAL_Init();
SystemClock_Config();
MX_GPIO_Init();
MX_USART1_UART_Init();
MX_I2C1_Init();
MPU6050_Init();
while (1) {
MPU6050_Read_Accel(&ax, &ay, &az);
// 对ax和ay进行高通滤波
ax_hpf = HighPassFilter(ax, ax_hpf);
ay_hpf = HighPassFilter(ay, ay_hpf);
if (abs(ax_hpf) > ACCEL_THRESHOLD || abs(ay_hpf) > ACCEL_THRESHOLD) {
// 如果加速度超过阈值,则输出到串口
char buffer[50];
sprintf(buffer, "ax: %d, ay: %d\r\n", ax_hpf, ay_hpf);
HAL_UART_Transmit(&huart1, (uint8_t *)buffer, strlen(buffer), HAL_MAX_DELAY);
}
HAL_Delay(10);
}
}
// 系统时钟配置
void SystemClock_Config(void) {
RCC_OscInitTypeDef RCC_OscInitStruct = {0};
RCC_ClkInitTypeDef RCC_ClkInitStruct = {0};
/** Configure the main internal regulator output voltage
*/
__HAL_RCC_PWR_CLK_ENABLE();
__HAL_PWR_VOLTAGESCALING_CONFIG(PWR_REGULATOR_VOLTAGE_SCALE1);
/** Initializes the RCC Oscillators according to the specified parameters
* in the RCC_OscInitTypeDef structure.
*/
RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSI;
RCC_OscInitStruct.HSIState = RCC_HSI_ON;
RCC_OscInitStruct.HSICalibrationValue = RCC_HSICALIBRATION_DEFAULT;
RCC_OscInitStruct.PLL.PLLState = RCC_PLL_ON;
RCC_OscInitStruct.PLL.PLLSource = RCC_PLLSOURCE_HSI_DIV2;
RCC_OscInitStruct.PLL.PLLMUL = RCC_PLL_MUL16;
if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK) {
Error_Handler();
}
/** Initializes the CPU, AHB and APB buses clocks
*/
RCC_ClkInitStruct.ClockType = RCC_CLOCKTYPE_HCLK | RCC_CLOCKTYPE_SYSCLK
| RCC_CLOCKTYPE_PCLK1 | RCC_CLOCKTYPE_PCLK2;
RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_PLLCLK;
RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1;
RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV2;
RCC_ClkInitStruct.APB2CLKDivider = RCC_HCLK_DIV1;
if (HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_2) != HAL_OK) {
Error_Handler();
}
}
// GPIO初始化
static void MX_GPIO_Init(void) {
GPIO_InitTypeDef GPIO_InitStruct = {0};
/* GPIO Ports Clock Enable */
__HAL_RCC_GPIOD_CLK_ENABLE();
__HAL_RCC_GPIOA_CLK_ENABLE();
/*Configure GPIO pin Output Level */
HAL_GPIO_WritePin(GPIOD, GPIO_PIN_12, GPIO_PIN_RESET);
/*Configure GPIO pin : PD12 */
GPIO_InitStruct.Pin = GPIO_PIN_12;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(GPIOD, &GPIO_InitStruct);
}
// USART1初始化
static void MX_USART1_UART_Init(void) {
huart1.Instance = USART1;
huart1.Init.BaudRate = 115200;
huart1.Init.WordLength = UART_WORDLENGTH_8B;
huart1.Init.StopBits = UART_STOPBITS_1;
huart1.Init.Parity = UART_PARITY_NONE;
huart1.Init.Mode = UART_MODE_TX_RX;
huart1.Init.HwFlowCtl = UART_HWCONTROL_NONE;
huart1.Init.OverSampling = UART_OVERSAMPLING_16;
if (HAL_UART_Init(&huart1) != HAL_OK) {
Error_Handler();
}
}
// I2C1初始化
void MX_I2C1_Init(void) {
hi2c1.Instance = I2C1;
hi2c1.Init.ClockSpeed = 400000;
hi2c1.Init.DutyCycle = I2C_DUTYCYCLE_2;
hi2c1.Init.OwnAddress1 = 0;
hi2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT;
hi2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE;
hi2c1.Init.OwnAddress2 = 0;
hi2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE;
hi2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE;
if (HAL_I2C_Init(&hi2c1) != HAL_OK) {
Error_Handler();
}
}
// 错误处理
void Error_Handler(void) {
__disable_irq();
while (1) {
}
}
```
需要根据您的具体情况进行修改,比如I2C通信的初始化需要根据您的硬件接口进行配置。当然,这里的高通滤波只是一种简单的实现,您可以根据实际应用场景进行优化。
阅读全文
相关推荐
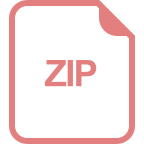
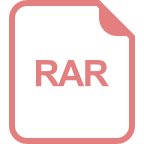
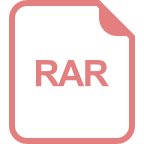
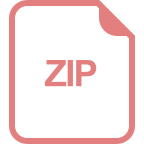
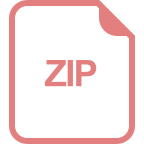
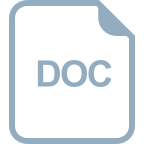
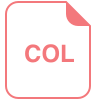
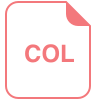





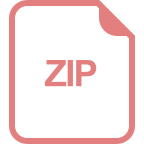
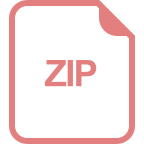
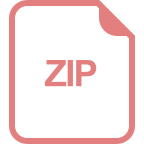