在硬件方面,需要使用STM32F103微控制器和光电对管,将光电对管的输出连接到PA0引脚。同时,需要连接一个绿灯和一个红灯,绿灯连接到PE0引脚,红灯连接到PE8引脚。最后,需要连接一个计算机,用于发送命令和接收故障信息。设计代码
时间: 2024-03-23 07:39:45 浏览: 55
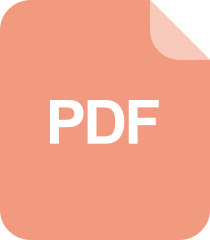
基于光电管的飞思卡尔智能车模自动循线控制系统
以下是基于STM32F103微控制器和uCOSIII操作系统的故障检测系统的设计代码:
```c
#include "stm32f10x.h"
#include "ucos_ii.h"
#include "usart.h"
#define TASK1_PRIORITY 5
#define TASK2_PRIORITY 6
#define TASK1_STACK_SIZE 128
#define TASK2_STACK_SIZE 128
#define LED_GREEN_PIN GPIO_Pin_0
#define LED_GREEN_PORT GPIOE
#define LED_RED_PIN GPIO_Pin_8
#define LED_RED_PORT GPIOE
#define SENSOR_PIN GPIO_Pin_0
#define SENSOR_PORT GPIOA
static OS_STK Task1Stack[TASK1_STACK_SIZE];
static OS_STK Task2Stack[TASK2_STACK_SIZE];
static OS_EVENT *semaphore;
static void Task1(void *pdata);
static void Task2(void *pdata);
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOE, ENABLE);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Pin = SENSOR_PIN;
GPIO_Init(SENSOR_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Pin = LED_GREEN_PIN | LED_RED_PIN;
GPIO_Init(LED_GREEN_PORT, &GPIO_InitStructure);
}
void USART_Configuration(void)
{
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART_SendString(char *str)
{
while (*str)
{
USART_SendData(USART1, *str++);
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
}
}
void USART_ReceiveString(char *str, uint16_t size)
{
uint16_t i = 0;
char c = 0;
while (i < size)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
c = USART_ReceiveData(USART1);
if (c == '\r' || c == '\n')
{
break;
}
*str++ = c;
i++;
}
*str = '\0';
}
void App_TaskCreate(void)
{
OSTaskCreate(Task1, (void *)0, &Task1Stack[TASK1_STACK_SIZE - 1], TASK1_PRIORITY);
OSTaskCreate(Task2, (void *)0, &Task2Stack[TASK2_STACK_SIZE - 1], TASK2_PRIORITY);
}
int main(void)
{
OSInit();
semaphore = OSSemCreate(0);
GPIO_Configuration();
USART_Configuration();
App_TaskCreate();
OSStart();
while (1);
}
static void Task1(void *pdata)
{
char command[12] = {0};
while (1)
{
USART_ReceiveString(command, 12);
if (strcmp(command, "202100171070") == 0)
{
GPIO_SetBits(LED_GREEN_PORT, LED_GREEN_PIN);
OSSemPost(semaphore);
}
}
}
static void Task2(void *pdata)
{
INT8U err;
int prev_state = 1;
int curr_state = 1;
char message[6] = {0};
while (1)
{
OSSemPend(semaphore, 0, &err);
curr_state = GPIO_ReadInputDataBit(SENSOR_PORT, SENSOR_PIN);
if (curr_state != prev_state)
{
if (curr_state == 0)
{
GPIO_ResetBits(LED_GREEN_PORT, LED_GREEN_PIN);
GPIO_SetBits(LED_RED_PORT, LED_RED_PIN);
USART_SendString("5a 83 52\r\n");
}
else
{
GPIO_ResetBits(LED_RED_PORT, LED_RED_PIN);
USART_SendString("5a 83 96\r\n");
}
prev_state = curr_state;
}
}
}
```
以上是该故障检测系统的设计代码,包括GPIO口和串口的初始化、任务的创建、中断服务程序的实现等。在任务1中,通过串口接收计算机发送的命令,并对命令进行解析;在任务2中,不断地检测光电对管的状态,并在检测到故障时点亮红灯,并向计算机发送故障信息。
阅读全文
相关推荐
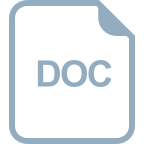
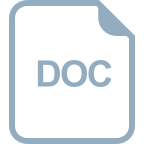





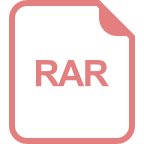
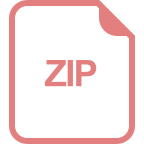
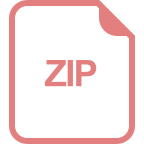
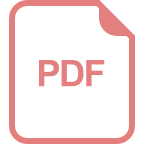
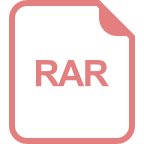
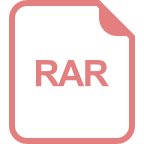
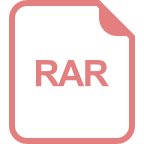
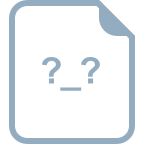
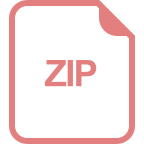
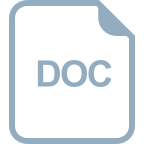
