解释一下struct Node { int line = 0; string type; string word; };
时间: 2024-05-28 09:11:21 浏览: 57
这是一个结构体的定义,结构体名为Node,包括三个成员变量line、type和word。
其中,line是一个整型变量,初始化为0;type是一个字符串类型的变量;word也是一个字符串类型的变量。
这个结构体可以用来表示一个文本文件中的单词,其中line表示单词所在的行数,type表示单词的类型(比如名词、动词等),word表示单词本身的内容。通过定义这样的结构体,可以方便地对文件中的内容进行处理和分析。
相关问题
C++中,譬如我设定了一个student类,里面有int age,string name,string Num,并且我已经将他们通过链表类串联,并且txt文件写入了student.txt中,那么比如我存了一些数据,我要指定修改某一个对象的age值再放回文件,我该怎么操作
要修改 student.txt 文件中某个学生对象的 age 值,可以先读取该文件中的所有学生对象数据,然后找到需要修改的学生对象,将其 age 值修改后再重新写入到 student.txt 文件中。以下是一个示例代码,可以实现该功能:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Student {
public:
Student(int age, string name, string num) : age(age), name(name), num(num) {}
int getAge() const {
return age;
}
void setAge(int age) {
this->age = age;
}
string getName() const {
return name;
}
void setName(string name) {
this->name = name;
}
string getNum() const {
return num;
}
void setNum(string num) {
this->num = num;
}
void writeToTxt(ofstream& file) const {
file << age << " " << name << " " << num << endl;
}
private:
int age;
string name;
string num;
};
class LinkedList {
public:
LinkedList() : head(nullptr) {}
void add(Student* student) {
Node* newNode = new Node;
newNode->student = student;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
} else {
Node* temp = head;
while (temp->next != nullptr) {
temp = temp->next;
}
temp->next = newNode;
}
}
void display() const {
Node* temp = head;
while (temp != nullptr) {
cout << "Name: " << temp->student->getName() << ", Age: " << temp->student->getAge() << ", Num: " << temp->student->getNum() << endl;
temp = temp->next;
}
}
void writeToTxt(string fileName) const {
ofstream file(fileName);
Node* temp = head;
while (temp != nullptr) {
temp->student->writeToTxt(file);
temp = temp->next;
}
file.close();
}
void modifyAge(string num, int newAge) {
Node* temp = head;
while (temp != nullptr) {
if (temp->student->getNum() == num) {
temp->student->setAge(newAge);
break;
}
temp = temp->next;
}
}
~LinkedList() {
Node* temp = head;
while (temp != nullptr) {
Node* next = temp->next;
delete temp->student;
delete temp;
temp = next;
}
}
private:
struct Node {
Student* student;
Node* next;
};
Node* head;
};
LinkedList* readFromTxt(string fileName) {
LinkedList* list = new LinkedList();
ifstream file(fileName);
if (file.is_open()) {
string line;
while (getline(file, line)) {
int age;
string name, num;
stringstream ss(line);
ss >> age >> name >> num;
Student* student = new Student(age, name, num);
list->add(student);
}
file.close();
} else {
cout << "Unable to open file " << fileName << endl;
}
return list;
}
int main() {
LinkedList* list = readFromTxt("student.txt");
cout << "Before modification:" << endl;
list->display();
list->modifyAge("001", 20);
cout << "After modification:" << endl;
list->display();
list->writeToTxt("student.txt");
delete list;
return 0;
}
```
在以上示例代码中,我们定义了一个 Student 类和一个 LinkedList 类。Student 类中包含了 age、name 和 num 三个属性,并提供了读取和修改这些属性的方法以及将该对象写入 txt 文件的方法。LinkedList 类中包含了添加学生对象、展示学生对象、将学生对象写入 txt 文件和修改学生对象 age 值的方法。在 main 函数中,我们首先调用 readFromTxt 方法读取 student.txt 文件中的所有学生对象数据,并将其存储到 LinkedList 对象中。然后,我们展示了所有学生对象的信息,并调用 modifyAge 方法修改了学号为 "001" 的学生对象的 age 值。最后,我们将修改后的学生对象数据重新写入到 student.txt 文件中。需要注意的是,在读取文件时,需要使用 ifstream 类型的对象,并使用 getline 函数逐行读取文件中的内容。同时,在修改数据时,需要遍历链表找到需要修改的学生对象,并调用其 setAge 方法修改其 age 值。
班主任朱老师喜欢在微信群中发布通知,让家长们接龙。某天朱老师发布了一则通知,“清明节放假三天,收到请回复”,于是家长们开始接龙: 1.png 但某个家长接龙时突然手滑了一下,回复成:“收到,猪老师”,于是后面的家长进行复制粘贴,也回复了“收到,猪老师",接龙变成了这样: 2.png 突然某个家长发现接错了,于是重新进行了接龙(当然,已经接错的是无法删除的),接龙终于又正常了: 3.png 结果很明显,当朱老师统计接龙信息时,他很生气! 输入格式: 第一行给出家长人数N(N<=100000)于是给家长编号为1~N,接下来若干行给出家长的接龙信息,每行表示一条接龙信息,格式为:收到,朱老师 家长编号,家长编号前有一个空格,接龙的最后一行为#,表示接龙结束,题目假设第一条接龙信息总是正确的。 输出格式: 在第一行中输出首个接错龙的家长编号;第二行按升序输出接错龙的家长编号,编号间用一个空格分隔,最后一个编号后面无空格,注意改正错误的家长不能被统计在里面;第三行按升序输出未接龙家长的编号,编号间用一个空格分隔,最后一个编号后面无空格。若没有家长接龙,输出"no one!";若没有家长接错龙,第二行输出"all right!",若所有家长都参与了接龙,第三行输出"everyone is here!" 用c语言完成
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Node {
char name[10];
int id;
struct Node* next;
};
struct Node* createNode(char name[], int id) {
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
strcpy(node->name, name);
node->id = id;
node->next = NULL;
return node;
}
struct Node* insertNode(struct Node* head, char name[], int id) {
struct Node* node = createNode(name, id);
if (head == NULL) {
return node;
}
struct Node* p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = node;
return head;
}
int main() {
int n;
scanf("%d", &n);
struct Node* head = NULL;
for (int i = 1; i <= n; i++) {
char name[10];
int id;
scanf("%s %*c%d", name, &id);
head = insertNode(head, name, id);
}
char line[20];
fgets(line, 20, stdin); // 读取接龙信息中的空行
int wrongId = 0;
struct Node* wrongHead = NULL;
struct Node* notJoinHead = NULL;
int joinCount = 0;
int* joinList = (int*)malloc(n * sizeof(int));
for (int i = 1; ; i++) {
fgets(line, 20, stdin);
if (line[0] == '#') {
break;
}
char name[10];
int id;
sscanf(line, "%*s %s %d", name, &id);
if (id != i + 1) {
if (wrongId == 0) {
wrongId = id;
}
wrongHead = insertNode(wrongHead, name, id);
}
joinList[joinCount++] = id;
}
if (wrongId == 0) {
printf("all right!\n");
} else {
printf("%d\n", wrongId);
struct Node* p = wrongHead;
while (p != NULL) {
printf("%d%c", p->id, (p->next == NULL ? '\n' : ' '));
p = p->next;
}
}
if (joinCount == n) {
printf("everyone is here!\n");
} else {
for (int i = 1; i <= n; i++) {
int found = 0;
for (int j = 0; j < joinCount; j++) {
if (joinList[j] == i) {
found = 1;
break;
}
}
if (!found) {
notJoinHead = insertNode(notJoinHead, "", i);
}
}
if (notJoinHead == NULL) {
printf("no one!\n");
} else {
struct Node* p = notJoinHead;
while (p != NULL) {
printf("%d%c", p->id, (p->next == NULL ? '\n' : ' '));
p = p->next;
}
}
}
return 0;
}
```
阅读全文
相关推荐
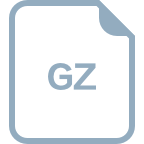















