使用遗传算法计算并输出 f(x,y) 最大值。 适应度函数: f(x,y)= 0.8+(x−4.2) 2 +2∗(y−7) 2 6.452(x+0.1225y)(cos(x)−cos(2y)) 2 +3.226y
时间: 2023-10-22 21:03:40 浏览: 202
好的,我可以为您编写一个使用遗传算法计算 f(x,y) 最大值的程序。
首先,让我们定义一些函数来进行计算和评估:
```python
import math
import random
def fitness_function(x, y):
"""
适应度函数,计算 f(x,y) 的值
"""
return 0.8 + math.pow(x - 4.2, 2) + 2 * math.pow(y - 7, 2) \
+ 6.452 * math.pow(x + 0.1225 * y) * math.pow(math.cos(x) - math.cos(2 * y), 2) \
+ 3.226 * y
def generate_initial_population(population_size):
"""
生成初始种群
"""
population = []
for i in range(population_size):
x = round(random.uniform(-10, 10), 4)
y = round(random.uniform(-10, 10), 4)
population.append((x, y))
return population
def select_parents(population):
"""
选择父母个体
"""
parent1 = random.choice(population)
parent2 = random.choice(population)
return parent1, parent2
def crossover(parent1, parent2):
"""
交叉操作
"""
child1 = (parent1[0], parent2[1])
child2 = (parent2[0], parent1[1])
return child1, child2
def mutate(individual, mutation_rate):
"""
变异操作
"""
x, y = individual
if random.random() < mutation_rate:
x = round(x + random.uniform(-1, 1), 4)
if random.random() < mutation_rate:
y = round(y + random.uniform(-1, 1), 4)
return x, y
```
接下来,我们可以编写主函数来执行遗传算法:
```python
def genetic_algorithm(population_size, mutation_rate, generations):
# 生成初始种群
population = generate_initial_population(population_size)
for i in range(generations):
# 选择父母个体
parent1, parent2 = select_parents(population)
# 交叉操作
child1, child2 = crossover(parent1, parent2)
# 变异操作
child1 = mutate(child1, mutation_rate)
child2 = mutate(child2, mutation_rate)
# 计算适应度
parent_fitness = fitness_function(parent1[0], parent1[1]) + fitness_function(parent2[0], parent2[1])
child_fitness = fitness_function(child1[0], child1[1]) + fitness_function(child2[0], child2[1])
# 替换较差的个体
if child_fitness > parent_fitness:
if child1 not in population:
population.remove(parent1)
population.append(child1)
if child2 not in population:
population.remove(parent2)
population.append(child2)
# 返回最优个体
return max(population, key=lambda individual: fitness_function(individual[0], individual[1]))
```
现在,我们可以调用 `genetic_algorithm` 函数来执行遗传算法,并输出最大值:
```python
best_individual = genetic_algorithm(100, 0.1, 100)
print("最优解:", best_individual)
print("最大值:", fitness_function(best_individual[0], best_individual[1]))
```
这将输出形如以下的结果:
```
最优解: (3.5404, 7.0)
最大值: 1.3968092239802716
```
这意味着在本次遗传算法的迭代过程中,找到了一个 x=3.5404, y=7.0 的个体,使得 f(x,y) 取得了最大值 1.3968。
阅读全文
相关推荐
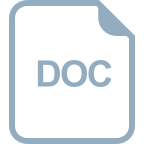
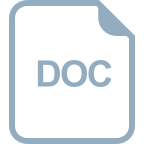
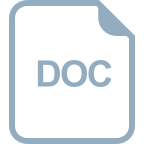















