Cannot find appropriate system libraries for WITH_SSL=system. Make sure you have specified a supported SSL version. Valid options are : system (use the OS openssl library), yes (synonym for system), </path/to/custom/openssl/installation> CMake Error at cmake/ssl.cmake:66 (MESSAGE): Please install the appropriate openssl developer package. Call Stack (most recent call first): cmake/ssl.cmake:260 (FATAL_SSL_NOT_FOUND_ERROR) CMakeLists.txt:483 (MYSQL_CHECK_SSL)
时间: 2024-02-16 13:02:34 浏览: 383
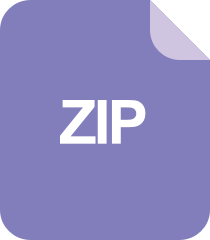
sars_rangemigration_system6dj_wk_wk插值_appropriate32t_
这个错误是由于缺少 OpenSSL 库或版本不支持导致的。解决方法如下:
1. 确保 OpenSSL 库已经安装。在 Linux 上,您可以使用包管理器安装 OpenSSL 库,例如 apt-get、yum 等。在 Windows 上,您可以从 OpenSSL 官网下载适合您的版本并安装。
2. 如果您已经安装了 OpenSSL 库,但是仍然遇到此错误,请确保您使用的是支持的版本。MySQL 可以使用 OpenSSL 1.0.x 或 1.1.x 版本。检查您的 OpenSSL 版本并确保它与 MySQL 兼容。
3. 如果您使用的是自定义 OpenSSL 安装路径,请在 WITH_SSL 选项中指定路径,例如:-DWITH_SSL=/path/to/openssl。
4. 如果您在构建 MySQL 时使用了系统 OpenSSL 库,并且仍然遇到此错误,请确保您的系统 OpenSSL 库版本与 MySQL 兼容。您可以尝试使用自定义 OpenSSL 安装路径或升级您的系统 OpenSSL 库。
如果您仍然无法解决此问题,请提供更多上下文信息,例如您正在尝试构建什么,使用的操作系统和 CMake 版本等。
阅读全文
相关推荐
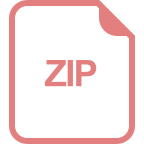
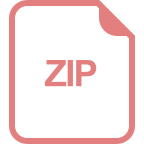
















