用c语言自定义递归函数,求1+2+3+……+n。
时间: 2023-10-07 12:10:35 浏览: 159
可以使用以下代码来自定义递归函数实现求和:
```c
#include <stdio.h>
int sum(int n) {
if (n == 1) {
return 1;
} else {
return n + sum(n - 1);
}
}
int main() {
int n;
printf("请输入一个正整数 n:");
scanf("%d", &n);
printf("1+2+3+...+%d=%d\n", n, sum(n));
return 0;
}
```
首先定义一个 `sum` 函数,接收一个整数 `n` 作为参数,如果 `n` 等于 1,直接返回 1,否则返回 `n` 和 `n-1` 的和,其中 `n-1` 通过递归调用 `sum` 函数得到。在 `main` 函数中,读入一个整数 `n`,并输出 `1+2+3+...+n` 的结果。
相关问题
使用c语言自定义递归函数,求1+2+3+……+n。
可以使用如下的递归函数来求解:
```c
int sum(int n) {
if (n == 1) {
return 1;
} else {
return n + sum(n-1);
}
}
```
这个函数的思路是:当 n 等于 1 的时候,返回 1;否则返回 n 加上 sum(n-1) 的结果,也就是 n 加上前面所有数的和。这样不断递归下去,最终就可以得到 1+2+3+...+n 的结果。
下面是一个完整的例子:
```c
#include <stdio.h>
int sum(int n);
int main() {
int n = 10;
int result = sum(n);
printf("1+2+3+...+%d = %d\n", n, result);
return 0;
}
int sum(int n) {
if (n == 1) {
return 1;
} else {
return n + sum(n-1);
}
}
```
输出结果为:
```
1+2+3+...+10 = 55
```
用C语言自定义递归函数,求1+2+3+……+n。
可以使用以下代码实现:
```c
#include <stdio.h>
int sum(int n) {
if (n == 1) {
return 1;
} else {
return n + sum(n - 1);
}
}
int main() {
int n;
printf("请输入一个正整数n:");
scanf("%d", &n);
printf("1+2+...+%d=%d\n", n, sum(n));
return 0;
}
```
首先定义了一个递归函数 `sum`,它的参数为整数 `n`,表示求 1 到 n 的累加和。如果 n 等于 1,则返回 1;否则返回 n 加上 sum(n-1) 的结果,即 1 到 n-1 的累加和再加上 n。
在主函数中,要求用户输入一个正整数 n,然后调用 `sum` 函数求出 1 到 n 的累加和,并输出结果。
阅读全文
相关推荐





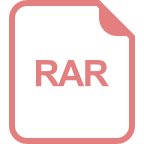




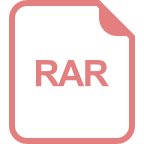



