softmax layer 代码
时间: 2023-07-02 22:19:11 浏览: 136
以下是使用Python实现softmax layer的示例代码:
```python
import numpy as np
def softmax(x):
"""
计算softmax函数的值
Args:
x: numpy数组,形状为(1, n),n为向量的维度
Returns:
s: numpy数组,形状为(1, n),softmax函数的值
"""
exp_x = np.exp(x)
s = exp_x / np.sum(exp_x, axis=1, keepdims=True)
return s
```
该函数接收一个形状为(1, n)的numpy数组,其中n为向量的维度,返回一个形状也为(1, n)的numpy数组,表示softmax函数的值。其中,np.exp(x)计算每个元素的指数值,np.sum(exp_x, axis=1, keepdims=True)计算所有元素的和,并将结果保留为二维数组。最后将每个元素除以总和,得到softmax函数的值。
相关问题
softmaxLayer
Softmax层是深度学习中常用的一种层类型,它主要用于解决多分类问题。在Softmax层中,对于输入的一组数据,该层会先对其进行指数映射,然后再根据每个元素相对于其他元素的大小来计算其在每个类别上的概率,输出的结果是一个概率分布。具体实现可以参考下面的范例代码:
```python
class SoftmaxLayer(Layer):
def softmax(x):
# 防止数值溢出
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum()
def forward(self, x):
self.x = x
out = SoftmaxLayer.softmax(x)
return out
def backward(self, dout):
dx = dout * SoftmaxLayer.softmax(self.x) * (1 - SoftmaxLayer.softmax(self.x))
return dx
```
在上面的代码中,forward函数实现了Softmax的前向传播,其中self.x保存了输入的数据。backward函数实现了Softmax的反向传播,其中dout是由后一层传递到当前层的梯度,dx表示传递到前一层的梯度。需要注意的是,在反向传播时Softmax层并没有向正向传播时借用SoftmaxWithLoss层实现一部分,而是一手全部包办了。因此SoftmaxLayer::Backward_cpu()函数也就被闲置了。[引用1][^1]
softmaxLayer的python代码和测试
softmaxLayer是一个常用的神经网络层,用于多分类问题中将原始输出转化为概率分布。其公式如下:
$$y_i = \frac{e^{x_i}}{\sum_{j=1}^{n} e^{x_j}}$$
其中,$x_i$表示原始输出的第$i$个元素,$y_i$表示通过softmax函数计算后的第$i$个输出元素。
以下是softmaxLayer的Python代码和测试:
```python
import numpy as np
class SoftmaxLayer:
def __init__(self):
self.output = None
def forward(self, input):
# 对每个样本进行softmax计算
exp_input = np.exp(input - np.max(input, axis=1, keepdims=True))
self.output = exp_input / np.sum(exp_input, axis=1, keepdims=True)
return self.output
def backward(self, input, grad_output, learning_rate):
# 计算softmax的梯度
softmax_grad = self.output * (1 - self.output)
# 计算损失函数对输入的梯度
grad_input = grad_output * softmax_grad
return grad_input
```
测试代码:
```python
np.random.seed(0)
# 构造输入和梯度
input = np.random.randint(0, 10, size=(3, 5))
grad_output = np.random.randn(3, 5)
# 创建Softmax层
softmax_layer = SoftmaxLayer()
# 前向传播
output = softmax_layer.forward(input)
print("Softmax层前向传播输出:")
print(output)
# 反向传播
grad_input = softmax_layer.backward(input, grad_output, 0.1)
print("Softmax层反向传播输出:")
print(grad_input)
```
阅读全文
相关推荐
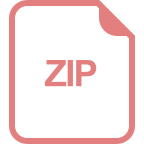
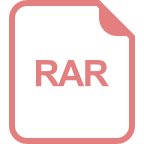
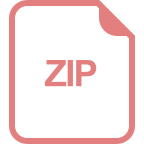












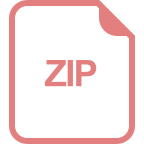