ROS 中C++ while(ros::ok())加上时间延时
时间: 2024-02-21 20:57:15 浏览: 39
在ROS中,可以使用 `ros::Rate` 类来实现循环执行代码并控制循环的频率。`ros::Rate` 类是ROS提供的一个方便的工具,用于控制程序的执行频率,其原理是通过计算当前时间与设定的时间间隔来控制循环的频率。
下面是一个简单的C++程序示例,其中包含了一个 `while(ros::ok())` 循环和时间延时的功能:
```cpp
#include <ros/ros.h>
int main(int argc, char **argv)
{
ros::init(argc, argv, "my_node");
ros::NodeHandle nh;
ros::Rate rate(10); // 设置循环频率为10Hz
while (ros::ok()) // 循环执行代码
{
// do some work
ROS_INFO("working...");
rate.sleep(); // 延时
}
return 0;
}
```
在上述程序中,`rate.sleep()` 函数用于控制程序的执行频率,其参数为时间间隔。在本例中,每个循环周期为 `1/10s`,即每秒循环10次。
如果需要执行一些比较耗时的任务,可以将任务放在 `while` 循环中,然后使用时间延时 `rate.sleep()` 来控制任务的执行频率,以避免程序负载过重导致崩溃。
如果需要调整程序的执行频率,只需要修改 `ros::Rate` 类的构造函数中的参数即可。
相关问题
ros中 while循环加上时间演示
在ROS中,可以使用定时器(`rospy.Timer()`)来实现定时执行某个函数的功能,同时也可以在函数中加入时间延时来控制函数执行的时间间隔。
下面是一个简单的 ROS 程序,其中包含了一个 `while` 循环和时间延时的功能:
```python
#!/usr/bin/env python
import rospy
import time
def main_loop():
while not rospy.is_shutdown():
# do some work
print("working...")
time.sleep(0.1) # time delay
if __name__ == '__main__':
rospy.init_node('my_node')
main_loop()
```
在上述程序中,`main_loop()` 函数是一个主循环,其中包含了一个 `while` 循环。在 `while` 循环中,程序每隔 `0.1` 秒输出一次 "working...",然后进行 `sleep` 延时。
在实际应用中,如果需要执行一些比较耗时的任务,可以将任务放在 `main_loop()` 函数中,然后使用时间延时来控制任务的执行频率,以避免程序负载过重导致崩溃。
同时,还可以使用 ROS 的定时器来实现类似的功能,如下所示:
```python
#!/usr/bin/env python
import rospy
def timer_callback(event):
print("working...")
if __name__ == '__main__':
rospy.init_node('my_node')
rospy.Timer(rospy.Duration(0.1), timer_callback)
rospy.spin()
```
在上述程序中,`timer_callback()` 函数是一个回调函数,用于处理定时器事件。在 `timer_callback()` 函数中,程序每隔 `0.1` 秒输出一次 "working..."。然后,使用 `rospy.Timer()` 函数来创建一个定时器,定时调用 `timer_callback()` 函数。最后,使用 `rospy.spin()` 函数来阻塞程序。
c++代码ros功能包中的ros::spin()的含义与作用
在 ROS 中,`ros::spin()` 是一个循环函数,主要用于处理 ROS 节点之间的消息通信,包括订阅消息、发布消息、服务调用等。在节点的 `main()` 函数中调用 `ros::spin()`,可以让节点一直运行,直到收到退出信号(如 Ctrl+C)才会退出。
`ros::spin()` 函数会进入 ROS 系统的事件循环,等待 ROS 节点之间的消息通信。当有消息到达时,`ros::spin()` 函数会调用对应的回调函数进行处理,处理完后继续等待下一条消息的到来。在处理消息的过程中,`ros::spin()` 函数不会占用 CPU 资源,因此可以在节点的主循环中使用。
总之,`ros::spin()` 是 ROS 节点之间消息通信的重要组成部分,它的作用是让节点一直运行,等待并处理 ROS 消息,保证节点的正常运行。
相关推荐
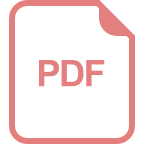
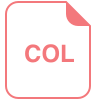
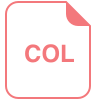
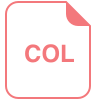









