stm32矩阵键盘代码
时间: 2023-09-10 19:08:39 浏览: 67
以下是一个简单的基于 STM32 的矩阵键盘代码,假设矩阵键盘为 4x4:
```c
#include "stm32f10x.h"
#define ROWS 4
#define COLUMNS 4
uint8_t row_pins[ROWS] = { GPIO_Pin_0, GPIO_Pin_1, GPIO_Pin_2, GPIO_Pin_3 };
uint8_t column_pins[COLUMNS] = { GPIO_Pin_4, GPIO_Pin_5, GPIO_Pin_6, GPIO_Pin_7 };
GPIO_TypeDef* row_ports[ROWS] = { GPIOA, GPIOA, GPIOA, GPIOA };
GPIO_TypeDef* column_ports[COLUMNS] = { GPIOA, GPIOA, GPIOA, GPIOA };
uint8_t key_map[ROWS][COLUMNS] = {
{ '1', '2', '3', 'A' },
{ '4', '5', '6', 'B' },
{ '7', '8', '9', 'C' },
{ '*', '0', '#', 'D' }
};
void init_gpio(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
char get_key(void) {
int row, column;
for (row = 0; row < ROWS; row++) {
GPIO_SetBits(row_ports[row], row_pins[row]);
for (column = 0; column < COLUMNS; column++) {
if (GPIO_ReadInputDataBit(column_ports[column], column_pins[column]) == 0) {
while (GPIO_ReadInputDataBit(column_ports[column], column_pins[column]) == 0);
GPIO_ResetBits(row_ports[row], row_pins[row]);
return key_map[row][column];
}
}
GPIO_ResetBits(row_ports[row], row_pins[row]);
}
return 0;
}
int main(void) {
char key;
init_gpio();
while (1) {
key = get_key();
if (key != 0) {
// Do something with key
}
}
}
```
代码中使用了 GPIO 控制矩阵键盘的行和列,然后根据行和列的状态来确定按下的按键。请根据自己的具体需求进行修改。
阅读全文
相关推荐










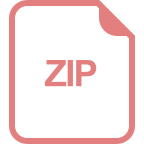


