用标准库写stm32矩阵键盘代码
时间: 2024-01-28 14:05:43 浏览: 28
以下是用标准库写的示例代码:
```c
#include "stm32f4xx.h"
#define ROW1 GPIO_Pin_0
#define ROW2 GPIO_Pin_1
#define ROW3 GPIO_Pin_2
#define ROW4 GPIO_Pin_3
#define COL1 GPIO_Pin_4
#define COL2 GPIO_Pin_5
#define COL3 GPIO_Pin_6
#define COL4 GPIO_Pin_7
#define KEYPAD_PORT GPIOE
void keypad_init(void) {
GPIO_InitTypeDef GPIO_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOE, ENABLE);
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_Pin = ROW1 | ROW2 | ROW3 | ROW4;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(KEYPAD_PORT, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStruct.GPIO_Pin = COL1 | COL2 | COL3 | COL4;
GPIO_Init(KEYPAD_PORT, &GPIO_InitStruct);
}
uint8_t keypad_scan(void) {
uint8_t row, col;
for (row = 0; row < 4; row++) {
GPIO_ResetBits(KEYPAD_PORT, ROW1 << row);
for (col = 0; col < 4; col++) {
if (!GPIO_ReadInputDataBit(KEYPAD_PORT, COL1 << col)) {
while (!GPIO_ReadInputDataBit(KEYPAD_PORT, COL1 << col));
return row * 4 + col + 1;
}
}
GPIO_SetBits(KEYPAD_PORT, ROW1 << row);
}
return 0;
}
int main(void) {
uint8_t key;
keypad_init();
while (1) {
key = keypad_scan();
if (key) {
// do something with the pressed key
}
}
}
```
该代码使用STM32F4的GPIO口模拟矩阵键盘,通过轮询的方式扫描键盘,返回被按下的键值。在主循环中不断扫描,当有键被按下时,执行相应的操作。
相关推荐








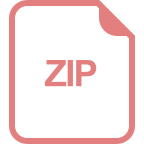