用c语言设计程序,输入一个中缀算术表达式,将其转换为后缀表达式并输出,然后对 后缀表达式进行求值。运算符包括“+”、“一”、“*”、“/”、“(”、“) 操作数为自然数,计算并输出其结果。 输入样例:125+(50*3-12)/10-26
时间: 2023-11-05 07:27:17 浏览: 45
好的,我可以为您解答这个问题。这是一个比较经典的算法题目,需要用到栈的数据结构来实现。下面是解题思路和代码示例:
1. 将中缀表达式转换为后缀表达式:
中缀表达式转后缀表达式的算法又称为 Shunting Yard 算法,其基本思想是利用一个栈来保存运算符,遇到数字直接输出,遇到运算符则将其与栈顶运算符进行比较,如果栈顶运算符优先级高于当前运算符则出栈输出,直到栈顶运算符优先级低于当前运算符或者栈为空,然后将当前运算符入栈。最终将栈中剩余运算符全部输出。
下面是 C 语言的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_SIZE 100
typedef struct {
int top;
char data[MAX_SIZE];
} Stack;
void InitStack(Stack *S) {
S->top = -1;
}
int Push(Stack *S, char c) {
if (S->top == MAX_SIZE - 1) {
return 0;
}
S->data[++S->top] = c;
return 1;
}
int Pop(Stack *S, char *c) {
if (S->top == -1) {
return 0;
}
*c = S->data[S->top--];
return 1;
}
int GetTop(Stack *S, char *c) {
if (S->top == -1) {
return 0;
}
*c = S->data[S->top];
return 1;
}
int IsEmpty(Stack *S) {
return S->top == -1;
}
int IsFull(Stack *S) {
return S->top == MAX_SIZE - 1;
}
int GetPriority(char c) {
switch (c) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
int InfixToPostfix(char *infix, char *postfix) {
Stack S;
InitStack(&S);
char c, tmp;
int i = 0, j = 0;
while ((c = infix[i++]) != '\0') {
if (isdigit(c)) {
postfix[j++] = c;
} else if (c == '(') {
Push(&S, c);
} else if (c == ')') {
while (Pop(&S, &tmp) && tmp != '(') {
postfix[j++] = tmp;
}
} else {
while (!IsEmpty(&S) && GetPriority(c) <= GetPriority(S.data[S.top])) {
Pop(&S, &tmp);
postfix[j++] = tmp;
}
Push(&S, c);
}
}
while (!IsEmpty(&S)) {
Pop(&S, &tmp);
postfix[j++] = tmp;
}
postfix[j] = '\0';
return 1;
}
```
2. 对后缀表达式进行求值:
后缀表达式求值的算法是利用一个栈来保存操作数,遇到数字直接入栈,遇到运算符则从栈中弹出两个操作数进行运算,然后将结果入栈。最终栈中剩余的元素即为表达式的值。
下面是 C 语言的代码示例:
```c
int PostfixEvaluate(char *postfix) {
Stack S;
InitStack(&S);
char c, tmp;
int i = 0, op1, op2, result;
while ((c = postfix[i++]) != '\0') {
if (isdigit(c)) {
Push(&S, c - '0');
} else {
Pop(&S, &op2);
Pop(&S, &op1);
switch (c) {
case '+':
result = op1 + op2;
break;
case '-':
result = op1 - op2;
break;
case '*':
result = op1 * op2;
break;
case '/':
result = op1 / op2;
break;
default:
return 0;
}
Push(&S, result);
}
}
Pop(&S, &result);
return result;
}
```
最后,将上面两个函数结合起来即可实现输入一个中缀算术表达式,将其转换为后缀表达式并输出,然后对后缀表达式进行求值,计算并输出其结果的功能。
完整的 C 语言代码如下:
相关推荐
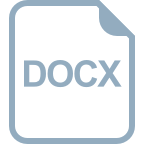
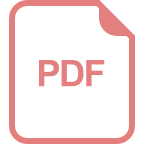
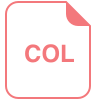
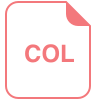












