用c语言设计一个非伪代码程序,将输人的中缀表达式转换成后缀表达式、然后将转换后的后缀表,达式进行计算(中级表达式只能输人个位数0~9和+,一,*,/四则运算)
时间: 2024-10-03 18:01:36 浏览: 40
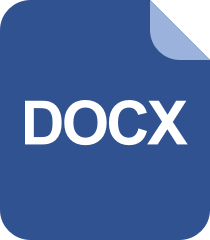
自定义栈中缀表达式转换为后缀表达式并求值
在 C 语言中,我们可以使用栈数据结构来实现中缀表达式到后缀表达式的转换(也称为逆波兰表示法),然后通过遍历后缀表达式并进行操作来求值。这里是一个简单的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_TOKENS 100
#define MAX_NUMBER 10
typedef struct {
char token[MAX_TOKENS];
int precedence;
} Operator;
void precedence(char op) {
switch (op) {
case '+': case '-':
return;
case '*': case '/':
return;
default:
printf("Invalid operator.\n");
exit(1);
}
}
bool isOperator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
void infix_to_postfix(char *infix, char *postfix) {
stack_t *stack = create_stack(MAX_TOKENS);
int i = 0, j = 0;
char op, ch;
while (infix[i]) {
if (isdigit(infix[i])) {
postfix[j++] = infix[i++];
postfix[j] = '\0';
} else if (isOperator(infix[i])) {
op = infix[i];
while (!stack->is_empty() && isOperator(stack->top.token[0]) && precedence(op) <= precedence(stack->top.token[0])) {
postfix[j++] = stack->pop().token[0];
}
stack->push({op, 0});
i++;
} else if (infix[i] == '(') {
stack->push({'(', 0});
i++;
} else if (infix[i] == ')') {
while (!stack->is_empty() && stack->top.token[0] != '(') {
postfix[j++] = stack->pop().token[0];
}
if (!stack->is_empty()) {
stack->pop(); // Pop the '('
}
i++;
}
}
while (!stack->is_empty()) {
postfix[j++] = stack->pop().token[0];
}
postfix[j] = '\0';
free_stack(stack);
}
int eval_postfix(char *postfix) {
stack_t *stack = create_stack(MAX_TOKENS);
char *token = strtok(postfix, " ");
double num1, num2, result;
while (token != NULL) {
if (isdigit(token[0])) {
num1 = atof(token);
stack->push({(double *) &num1, 0});
} else {
num2 = atof(stack->top.value); // Pop the previous number
switch (token[0]) {
case '+':
result = stack->top.value + num2; // Perform operation and push result back
break;
case '-':
result = stack->top.value - num2;
break;
case '*':
result = stack->top.value * num2;
break;
case '/':
if (num2 != 0)
result = stack->top.value / num2;
else {
printf("Error: Division by zero.\n");
return -1;
}
break;
default:
printf("Invalid operator.\n");
return -1;
}
stack->pop();
stack->push({(double *) &result, 0});
}
token = strtok(NULL, " ");
}
return stack->top.value;
}
int main() {
char infix[100], postfix[MAX_TOKENS + 1];
printf("Enter an arithmetic expression in infix notation: ");
fgets(infix, sizeof(infix), stdin);
infix[strlen(infix) - 1] = '\0'; // Remove newline character
printf("Converted to postfix: %s\n", postfix);
int result = eval_postfix(postfix);
if (result == -1) {
return -1;
} else {
printf("Result: %.2f\n", result);
}
return 0;
}
```
在这个程序中,我们首先定义了一个栈来存储操作符及其优先级,然后遍历输入的中缀表达式,按规则将其转换为后缀表达式。接着,我们遍历后缀表达式,并根据运算符进行相应的计算。
**相关问题--:**
1. 为什么要先处理左括号再处理运算符?
2. 如何改进这个程序以支持更复杂的算术表达式(如指数、括号内的运算等)?
3. 如果用户输入了非法字符,如何在程序中增加错误检查?
阅读全文
相关推荐
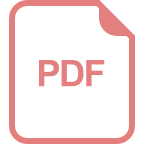
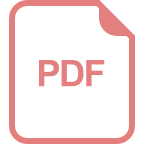










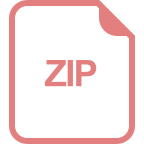
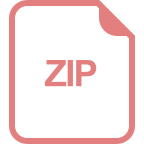
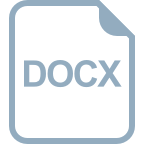
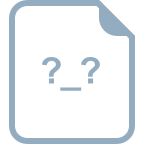
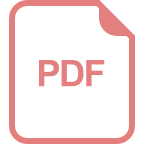
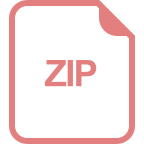
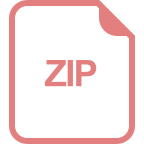