假设你是一个python开发工程师,你想在一个文件用asyncio启动了一个协程启动了tcp的client服务,这个服务包括连接tcp,发送tcp的消息并接收返回值这两个任务,这个文件的tcp需要保持一直在运行,又开辟了一个新的文件作为主函数,然后你想在主文件中调用这个协程的tcp发送消息,你该怎么做,能举个例子吗
时间: 2023-06-27 16:04:27 浏览: 46
首先,在一个文件中启动一个异步的TCP客户端可以这样实现:
```python
import asyncio
async def tcp_client():
reader, writer = await asyncio.open_connection('localhost', 8888)
while True:
message = input("Enter message to send: ")
writer.write(message.encode())
data = await reader.read(100)
print(f'Received: {data.decode()}')
async def main():
await tcp_client()
if __name__ == '__main__':
asyncio.run(main())
```
这个协程会一直运行,等待用户输入消息并发送,然后等待服务器返回数据并打印到控制台上。
然后,在另外一个文件中,我们可以通过调用`asyncio.run_coroutine_threadsafe()`方法来在主函数中调用TCP发送消息的协程。例如:
```python
import asyncio
from tcp_client import tcp_client
async def main():
loop = asyncio.get_event_loop()
future = asyncio.run_coroutine_threadsafe(tcp_client(), loop)
await asyncio.sleep(1) # wait for the connection to establish
# send a message through the TCP client
message = 'Hello, world!'
future.result().writer.write(message.encode())
if __name__ == '__main__':
asyncio.run(main())
```
这里我们先通过`asyncio.get_event_loop()`方法获取事件循环,然后通过`asyncio.run_coroutine_threadsafe()`方法在事件循环中执行TCP客户端协程,并获取一个`Future`对象。我们可以通过`future.result()`方法获取这个`Future`对象的返回值,也就是TCP客户端的`reader`和`writer`对象。然后就可以使用`writer.write()`方法向服务器发送消息了。注意,在发送消息前需要等待一段时间,让TCP连接成功建立,否则会出现连接拒绝的错误。这里我们通过`await asyncio.sleep(1)`方法等待了1秒钟。
相关推荐
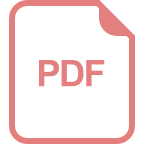
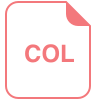
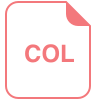
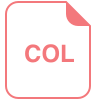
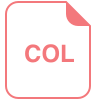









