keras获取graph_def
时间: 2023-09-03 20:08:15 浏览: 45
要在 Keras 中获取 graph_def,您可以使用以下代码:
```python
import tensorflow as tf
from keras import backend as K
sess = tf.keras.backend.get_session()
graph_def = sess.graph.as_graph_def()
```
这将返回一个包含 Keras 模型的图形定义的 TensorFlow GraphDef 对象。您可以使用 TensorFlow 提供的其他工具来进一步处理和分析此对象。
相关问题
tensorflow将pb文件转换为h5文件
可以使用TensorFlow提供的tf.compat.v1.keras.models.load_model()函数将pb文件转换为h5文件。具体步骤如下:
1. 加载pb文件
```python
import tensorflow as tf
# 加载pb文件
with tf.io.gfile.GFile('model.pb', "rb") as f:
graph_def = tf.compat.v1.GraphDef()
graph_def.ParseFromString(f.read())
```
2. 转换为keras模型
```python
# 转换为keras模型
with tf.compat.v1.Session() as sess:
tf.compat.v1.import_graph_def(graph_def, name='')
model = tf.compat.v1.keras.models.model_from_config(
sess.graph.as_graph_def().config)
```
3. 保存为h5文件
```python
# 保存为h5文件
model.save('model.h5')
```
完整代码示例:
```python
import tensorflow as tf
# 加载pb文件
with tf.io.gfile.GFile('model.pb', "rb") as f:
graph_def = tf.compat.v1.GraphDef()
graph_def.ParseFromString(f.read())
# 转换为keras模型
with tf.compat.v1.Session() as sess:
tf.compat.v1.import_graph_def(graph_def, name='')
model = tf.compat.v1.keras.models.model_from_config(
sess.graph.as_graph_def().config)
# 保存为h5文件
model.save('model.h5')
```
注意:在使用tf.compat.v1.keras.models.load_model()函数加载h5文件时,需要将custom_objects参数设置为相应的自定义层或损失函数。
在tensorflow1.1.4版本中出现cannot import name 'export_saved_model' from 'tensorflow.python.keras.saving.saved_model'的报错,该怎么办
在TensorFlow 1.1.4版本中,`export_saved_model`函数是没有实现的,因此无法从`tensorflow.python.keras.saving.saved_model`中导入。
如果您需要导出SavedModel,可以使用下面的代码:
```python
import tensorflow as tf
# Build and train your model
# Export the model as a SavedModel
with tf.Session(graph=tf.Graph()) as sess:
# Build the model graph
# ...
# Train the model
# ...
# Export the model as a SavedModel
builder = tf.saved_model.builder.SavedModelBuilder('/path/to/export/dir')
builder.add_meta_graph_and_variables(sess, [tf.saved_model.tag_constants.SERVING], signature_def_map={
'predict': predict_signature_def,
'train': train_signature_def,
# Add additional signature defs as needed
})
builder.save()
```
请注意,此代码是基于TensorFlow 1.1.4版本,如果您使用的是其他版本,则可能需要进行适当的修改。
相关推荐
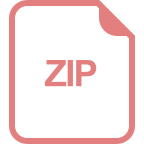












