PCL对点云进行平面拟合 代码
时间: 2023-09-11 16:07:18 浏览: 59
PCL(Point Cloud Library)是一个非常流行的点云处理库,其中包含了许多点云处理的算法和工具函数。下面是使用PCL对点云进行平面拟合的示例代码:
```cpp
#include <pcl/point_types.h>
#include <pcl/features/normal_3d.h>
#include <pcl/sample_consensus/method_types.h>
#include <pcl/sample_consensus/model_types.h>
#include <pcl/segmentation/sac_segmentation.h>
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
// Fill in the cloud data
cloud->width = 15;
cloud->height = 1;
cloud->points.resize (cloud->width * cloud->height);
for (size_t i = 0; i < cloud->points.size (); ++i)
{
cloud->points[i].x = 1024 * rand () / (RAND_MAX + 1.0f);
cloud->points[i].y = 1024 * rand () / (RAND_MAX + 1.0f);
cloud->points[i].z = 1.0;
}
// Create the normal estimation class, and pass the input dataset to it
pcl::NormalEstimation<pcl::PointXYZ, pcl::Normal> ne;
ne.setInputCloud (cloud);
// Create an empty kdtree representation, and pass it to the normal estimation object.
// Its content will be filled inside the object, based on the given input dataset (as no other search surface is given).
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree (new pcl::search::KdTree<pcl::PointXYZ> ());
ne.setSearchMethod (tree);
// Output datasets
pcl::PointCloud<pcl::Normal>::Ptr cloud_normals (new pcl::PointCloud<pcl::Normal>);
// Use all neighbors in a sphere of radius 3cm
ne.setRadiusSearch (0.03);
// Compute the features
ne.compute (*cloud_normals);
// Create the segmentation object for the planar model and set all the parameters
pcl::SACSegmentationFromNormals<pcl::PointXYZ, pcl::Normal> seg;
seg.setOptimizeCoefficients (true);
seg.setModelType (pcl::SACMODEL_PLANE);
seg.setMethodType (pcl::SAC_RANSAC);
seg.setMaxIterations (1000);
seg.setDistanceThreshold (0.01);
seg.setInputCloud (cloud);
seg.setInputNormals (cloud_normals);
// Obtain the plane inliers and coefficients
pcl::ModelCoefficients::Ptr coefficients (new pcl::ModelCoefficients);
pcl::PointIndices::Ptr inliers (new pcl::PointIndices);
seg.segment (*inliers, *coefficients);
// Print the model coefficients
std::cerr << "Model coefficients: " << coefficients->values[0] << " "
<< coefficients->values[1] << " "
<< coefficients->values[2] << " "
<< coefficients->values[3] << std::endl;
```
这段代码的主要作用是随机生成一个包含15个点的点云,然后使用PCL对点云进行平面拟合。具体步骤如下:
1. 创建一个PointCloud对象,并随机生成15个点。
2. 创建一个NormalEstimation对象,并设置输入点云和搜索方法。
3. 创建一个PointCloud对象,用于存储法线。
4. 设置法线估计的参数,并计算法线。
5. 创建一个SACSegmentationFromNormals对象,并设置模型类型、方法类型、最大迭代次数和距离阈值等参数。
6. 设置输入点云和法线,执行平面拟合。
7. 输出平面拟合的模型系数。
需要注意的是,这里使用的是SACSegmentationFromNormals算法,是基于法线的平面拟合,因此需要先计算法线。如果不需要计算法线,可以使用SACSegmentation算法。
相关推荐
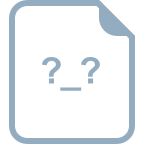














