PCL对点云数据进行平面拟合 代码
时间: 2023-11-22 13:05:42 浏览: 31
PCL(Point Cloud Library)是一个开源的点云数据处理库,其提供了很多点云数据处理的算法,包括平面拟合。
下面是一个简单的示例代码,展示了如何使用PCL对点云数据进行平面拟合:
```cpp
#include <iostream>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
#include <pcl/features/normal_3d.h>
#include <pcl/segmentation/sac_segmentation.h>
int main(int argc, char** argv)
{
// 读取点云数据
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
pcl::io::loadPCDFile<pcl::PointXYZ>("cloud.pcd", *cloud);
// 计算点云法向量
pcl::NormalEstimation<pcl::PointXYZ, pcl::Normal> ne;
ne.setInputCloud(cloud);
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>());
ne.setSearchMethod(tree);
pcl::PointCloud<pcl::Normal>::Ptr cloud_normals(new pcl::PointCloud<pcl::Normal>);
ne.setRadiusSearch(0.03);
ne.compute(*cloud_normals);
// 平面拟合
pcl::ModelCoefficients::Ptr coefficients(new pcl::ModelCoefficients);
pcl::PointIndices::Ptr inliers(new pcl::PointIndices);
pcl::SACSegmentationFromNormals<pcl::PointXYZ, pcl::Normal> seg;
seg.setOptimizeCoefficients(true);
seg.setModelType(pcl::SACMODEL_NORMAL_PLANE);
seg.setMethodType(pcl::SAC_RANSAC);
seg.setNormalDistanceWeight(0.1);
seg.setMaxIterations(100);
seg.setDistanceThreshold(0.03);
seg.setInputCloud(cloud);
seg.setInputNormals(cloud_normals);
seg.segment(*inliers, *coefficients);
// 输出平面参数
std::cout << "平面法向量:" << coefficients->values[0] << " "
<< coefficients->values[1] << " "
<< coefficients->values[2] << std::endl;
std::cout << "平面偏移量:" << coefficients->values[3] << std::endl;
return 0;
}
```
在这个示例中,我们读取了一个点云数据,并使用`NormalEstimation`计算了点云的法向量。然后,我们使用`SACSegmentationFromNormals`对点云数据进行平面拟合,输出了平面的法向量和偏移量。
需要注意的是,这只是一个简单的示例,实际的平面拟合应用中可能需要调整参数以得到更好的结果。
相关推荐
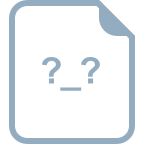














