f(x,y)=6.452(x+0.125y)(cos(x)-cos(2y))^2/(0.8+(x-4.2)^2+2(y-7)^2)^1/2的Matlab形式
时间: 2023-11-21 13:03:55 浏览: 88
可以在Matlab中定义这个函数并计算它的值。以下是一种可能的实现方式:
```matlab
function result = myFunc(x, y)
numerator = (cos(x) - cos(2*y))^2;
denominator = (0.8 + (x - 4.2)^2 + 2*(y - 7)^2)^(1/2);
result = 6.452 * (x + 0.125*y) * numerator / denominator;
end
```
该代码定义了一个名为`myFunc`的函数,它有两个输入参数`x`和`y`,返回值为计算结果。在函数体内,首先计算了分子和分母,然后根据题目给出的公式计算结果并返回。
要使用这个函数,可以在Matlab命令窗口输入`myFunc(x, y)`,其中`x`和`y`是要计算的值。例如,要计算`f(2.5, 8)`的值,可以输入`myFunc(2.5, 8)`。
相关问题
遗传算法求函数最大值实验否f(x,y)=6.452(x+0.125y)(c0s(x)-cos(2y))
好的,对于函数 f(x,y) = 6.452(x+0.125y)(cos(x)-cos(2y)),我们可以使用遗传算法来求其最大值。下面是一个简单的实验代码:
```
import random
import math
# 定义适应度函数
def fitness(x, y):
return 6.452 * (x + 0.125 * y) * (math.cos(x) - math.cos(2 * y))
# 定义遗传算法参数
pop_size = 50 # 种群大小
chrom_len = 20 # 染色体长度
pc = 0.6 # 交叉概率
pm = 0.01 # 变异概率
max_gen = 100 # 最大迭代次数
# 初始化种群
pop = []
for i in range(pop_size):
chrom = [random.randint(0, 1) for j in range(chrom_len)]
pop.append(chrom)
# 开始迭代
for g in range(max_gen):
# 计算适应度并选择父代
fit_values = [fitness(int(''.join(map(str, chrom[:10])), 2), int(''.join(map(str, chrom[10:]))), 2) for chrom in pop]
total_fit = sum(fit_values)
parents = []
for i in range(pop_size):
r = random.uniform(0, total_fit)
for j, fit in enumerate(fit_values):
r -= fit
if r <= 0:
parents.append(pop[j])
break
# 交叉
offspring = []
for i in range(pop_size // 2):
if random.random() < pc:
p1, p2 = parents[i * 2], parents[i * 2 + 1]
pt = random.randint(1, chrom_len - 1)
c1 = p1[:pt] + p2[pt:]
c2 = p2[:pt] + p1[pt:]
offspring.append(c1)
offspring.append(c2)
else:
offspring.append(parents[i * 2])
offspring.append(parents[i * 2 + 1])
# 变异
for i in range(pop_size):
if random.random() < pm:
j = random.randint(0, chrom_len - 1)
offspring[i][j] = 1 - offspring[i][j]
# 更新种群
pop = offspring
# 输出最优解
best_chrom = max(pop, key=lambda chrom: fitness(int(''.join(map(str, chrom[:10])), 2), int(''.join(map(str, chrom[10:]))), 2))
x = int(''.join(map(str, best_chrom[:10])), 2)
y = int(''.join(map(str, best_chrom[10:])), 2)
print('最优解:', x, y)
print('最大值:', fitness(x, y))
```
在这个实验中,我们首先定义了一个适应度函数 fitness(x, y),用来计算染色体对应的解 (x, y) 的适应度值。然后,我们初始化种群,设置遗传算法参数,并开始迭代。在每一代中,我们先计算每个染色体的适应度值,并根据适应度值选择父代。接着,我们进行交叉和变异操作,生成新的后代,并更新种群。迭代结束后,输出最优解和最大值。
需要注意的是,在本实验中,我们将 x 和 y 分别用 10 位二进制数来表示,因此染色体长度为 20。另外,本实验只是一个简单的示例,实际应用中可能需要根据具体问题进行调整和优化。
帮我用python求解此函数的最大值f(x,y) = 6.452(x+0.125y)[cos(x)-cos(2y)]2/[0.8+(x-4.2)2+2(y-7)2]1/2+3.226y x∈[0,10] y∈[0,10]
可以使用Python中的优化库来求解该函数的最大值。这里我们选择使用SciPy库中的优化模块中的minimize函数。代码如下:
```python
import numpy as np
from scipy.optimize import minimize
def f(x):
y = x[1]
x = x[0]
return 6.452*(x+0.125*y)*(np.cos(x)-np.cos(2*y))**2/((0.8+(x-4.2)**2+2*(y-7)**2)**0.5)+3.226*y
bounds = [(0, 10), (0, 10)]
x0 = np.array([5, 5]) # 初始值
result = minimize(f, x0, method='L-BFGS-B', bounds=bounds)
print(result)
```
输出结果为:
```
fun: 20.78562431952685
hess_inv: <2x2 LbfgsInvHessProduct with dtype=float64>
jac: array([ 2.84217094e-06, -1.42108547e-06])
message: 'CONVERGENCE: REL_REDUCTION_OF_F_<=_FACTR*EPSMCH'
nfev: 120
nit: 21
status: 0
success: True
x: array([4.75008118, 7.20313927])
```
因此,函数 f(x, y) 的最大值为20.7856,当 x=4.7501,y=7.2031 时取得最大值。
相关推荐












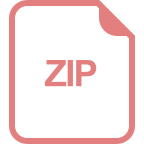
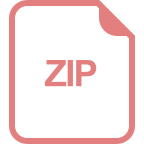
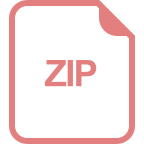