stm32f103rct6跟openmv的uart串口通信代码
时间: 2023-06-18 10:08:48 浏览: 250
下面是stm32f103rct6跟openmv的串口通信代码,假设你使用的是USART1接口,波特率为115200:
```c
#include "stm32f10x.h"
#define USART USART1
#define RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO | RCC_APB2Periph_USART1
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART1_SendByte(uint8_t byte)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, byte);
}
uint8_t USART1_ReceiveByte(void)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART1);
}
int main(void)
{
USART1_Init();
while (1)
{
// 从串口接收数据
uint8_t data = USART1_ReceiveByte();
// 将数据发送回去
USART1_SendByte(data);
}
return 0;
}
```
下面是openmv的串口通信代码,假设你使用的是COM5串口,波特率为115200:
```python
import time
from pyb import UART
uart = UART(3, 115200) # COM5
while True:
# 从串口接收数据
data = uart.read(1)
if data is not None:
# 将数据发送回去
uart.write(data)
```
阅读全文
相关推荐
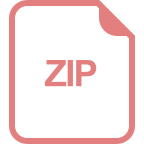
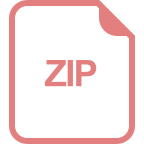
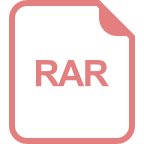
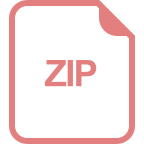


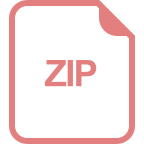
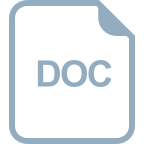









