使用C++进行编程,假设x和y是两个由单链表组成的字符串(其中每个结点只存储一个字符),请编写一个算法,找出x中第一个不在y中出现的字符。
时间: 2023-05-27 07:02:45 浏览: 238
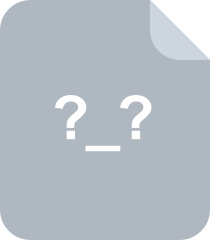
在一个字符串中找到第一个只出现一次的字符
思路:
1. 遍历链表x中的每个节点,对于每个节点,判断该节点的值(即字符)是否在链表y中出现过。
2. 如果该字符未在y中出现过,直接返回该字符即可。
3. 如果遍历完链表x仍未找到符合条件的字符,则返回空字符。
代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
//定义链表结点
typedef struct Node {
char data; //数据
struct Node* next; //指向下一个结点的指针
} Node;
//创建链表
Node* createList() {
Node *head, *tail; //定义头指针和尾指针
head = tail = NULL; //初始时头指针和尾指针都为NULL
char ch;
while ((ch = getchar()) != '\n') {
Node* newNode = (Node*) malloc(sizeof(Node)); //创建新结点
newNode->data = ch;
newNode->next = NULL;
if (head == NULL) { //如果链表为空,则新结点既是头结点也是尾结点
head = tail = newNode;
} else { //否则将新结点添加到链表尾部
tail->next = newNode;
tail = newNode;
}
}
return head;
}
//查找第一个不在y中出现的字符
char findChar(Node* x, Node* y) {
Node* px = x; //定义指向链表x的指针
while (px != NULL) {
Node* py = y; //定义指向链表y的指针
while (py != NULL) {
if (px->data == py->data) { //如果该字符在y中出现过,则跳出循环进行下一次比较
break;
}
py = py->next;
}
if (py == NULL) { //如果该字符未在y中出现过,则直接返回该字符
return px->data;
}
px = px->next;
}
return '\0'; //如果遍历完链表x未找到符合条件的字符,则返回空字符
}
int main() {
Node* x = createList(); //创建链表x
Node* y = createList(); //创建链表y
char c = findChar(x, y); //查找第一个不在y中出现的字符
if (c != '\0') {
printf("%c\n", c);
} else {
printf("Not found.\n");
}
return 0;
}
```
示例输入:
```
abcdefgh
acdg
```
示例输出:
```
b
```
阅读全文
相关推荐













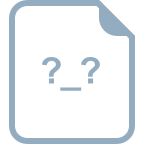
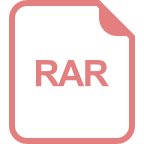
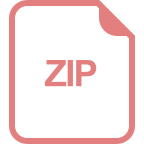