python自动搜索图片中的近似三角形,最后测量三角形的三个角大小,结果输出到B文件夹下
时间: 2024-06-09 17:07:53 浏览: 12
你可以使用Python中的OpenCV库来实现这个功能。以下是一个简单的步骤:
1. 从文件夹中读取图片并将其转换为灰度图像。
2. 使用Canny边缘检测算法查找图像中的边缘。
3. 使用霍夫变换检测图像中的直线,并根据线段的长度和角度过滤掉不需要的线段。
4. 对剩余的线段进行聚类,以找到三角形的三条边。
5. 计算三角形的三个角度,并将结果输出到B文件夹下。
下面是一个基本的代码示例:
```python
import cv2
import numpy as np
import math
import os
# 读取图片
img = cv2.imread('A/image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# 检测直线
lines = cv2.HoughLinesP(edges, 1, np.pi/180, 50, minLineLength=50, maxLineGap=5)
# 过滤掉不需要的线段
filtered_lines = []
for line in lines:
x1, y1, x2, y2 = line[0]
length = math.sqrt((x2 - x1)**2 + (y2 - y1)**2)
angle = math.atan2(y2 - y1, x2 - x1) * 180 / np.pi
if length > 50 and abs(angle) < 80:
filtered_lines.append(line[0])
# 聚类线段
clusters = cv2.groupRectangles(filtered_lines, 1, 0.2)
# 计算三个角的大小
for cluster in clusters[0]:
x1, y1, x2, y2 = cluster
for cluster2 in clusters[0]:
if np.array_equal(cluster, cluster2):
continue
x3, y3, x4, y4 = cluster2
for cluster3 in clusters[0]:
if np.array_equal(cluster, cluster3) or np.array_equal(cluster2, cluster3):
continue
x5, y5, x6, y6 = cluster3
# 计算三个角
a = math.sqrt((x2 - x1)**2 + (y2 - y1)**2)
b = math.sqrt((x4 - x3)**2 + (y4 - y3)**2)
c = math.sqrt((x6 - x5)**2 + (y6 - y5)**2)
A = math.degrees(math.acos((b**2 + c**2 - a**2) / (2 * b * c)))
B = math.degrees(math.acos((c**2 + a**2 - b**2) / (2 * c * a)))
C = math.degrees(math.acos((a**2 + b**2 - c**2) / (2 * a * b)))
print(A, B, C)
# 将结果输出到B文件夹下
if not os.path.exists('B'):
os.makedirs('B')
with open('B/angles.txt', 'a') as f:
f.write('{}, {}, {}\n'.format(A, B, C))
```
请注意,上面的代码只是一种基本的方法来实现这个功能。对于不同的图片和场景,您可能需要进行一些微调。
相关推荐
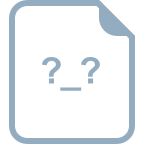















