6.编写程序,生成一个包含20个介于1到50之间随机整数的列表,然后使用插入法对给定整数列表中的所有元素升序排序。
时间: 2024-03-03 12:47:31 浏览: 40
好的,以下是Python代码实现:
```
import random
# 生成包含20个介于1到50之间随机整数的列表
nums = [random.randint(1, 50) for i in range(20)]
print("原始列表:", nums)
# 插入法排序
for i in range(1, len(nums)):
j = i
while j > 0 and nums[j] < nums[j-1]:
nums[j], nums[j-1] = nums[j-1], nums[j]
j -= 1
print("排序后的列表:", nums)
```
这个程序首先使用列表生成式生成包含20个介于1到50之间随机整数的列表。接着,使用插入法对给定整数列表中的所有元素升序排序。最后输出排序后的列表。
相关问题
编写程序,生成一个包含20个介于1到50之间随机整数的列表,然后使用插入法对给定整数列表中的所有元素升序排序。
以下是Python代码实现:
```python
import random
# 生成包含20个介于1到50之间随机整数的列表
random_list = [random.randint(1, 50) for _ in range(20)]
print("生成的随机整数列表为:", random_list)
# 使用插入法对列表中的所有元素升序排序
for i in range(1, len(random_list)):
key = random_list[i]
j = i - 1
while j >= 0 and key < random_list[j]:
random_list[j + 1] = random_list[j]
j -= 1
random_list[j + 1] = key
print("排序后的列表为:", random_list)
```
输出结果类似于:
```
生成的随机整数列表为: [7, 50, 45, 11, 22, 44, 1, 7, 14, 31, 15, 30, 23, 18, 16, 37, 31, 10, 40, 2]
排序后的列表为: [1, 2, 7, 7, 10, 11, 14, 15, 16, 18, 22, 23, 30, 31, 31, 37, 40, 44, 45, 50]
```
3.用户输入一个列表和2个整数作为下标,然后使用切片获取并输出列表中介于2个下标之间的元素组成的子列表。例如用户输入[1,2,3,4,5,6]和2,5,程序输出[3,4,5,6]。
好的,我可以帮你回答这个问题。实现的代码如下:
```python
lst = [1, 2, 3, 4, 5, 6]
start = 2
end = 5
sub_lst = lst[start:end+1]
print(sub_lst)
```
这段代码首先定义了一个列表`lst`,然后给定了起始下标`start`和终止下标`end`。接着,使用切片`lst[start:end+1]`获取介于这两个下标之间的元素组成的子列表,并将其保存到变量`sub_lst`中。最后,使用`print()`函数输出该子列表。这样就实现了获取并输出列表中介于两个下标之间的元素组成的子列表的功能。
相关推荐
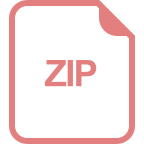












