c语言凯撒密码、字母倒排序
时间: 2023-12-01 15:04:09 浏览: 84
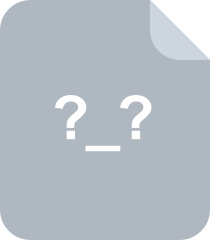
凯撒密码c语言 凯撒密码c语言
凯撒密码的实现可以使用 C 语言的字符数组和循环,可以将每个字符向后移动固定的步长,也可以将每个字符向前移动固定的步长,具体实现如下:
```c
#include <stdio.h>
#include <string.h>
void caesar_encrypt(char* str, int shift) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] >= 'a' && str[i] <= 'z') {
str[i] = (str[i] - 'a' + shift) % 26 + 'a';
} else if (str[i] >= 'A' && str[i] <= 'Z') {
str[i] = (str[i] - 'A' + shift) % 26 + 'A';
}
}
}
void caesar_decrypt(char* str, int shift) {
caesar_encrypt(str, 26 - shift);
}
int main() {
char message[] = "hello world";
int shift = 3;
printf("Original message: %s\n", message);
caesar_encrypt(message, shift);
printf("Encrypted message: %s\n", message);
caesar_decrypt(message, shift);
printf("Decrypted message: %s\n", message);
return 0;
}
```
其中,`caesar_encrypt` 函数将字符串中的字符向后移动 `shift` 个位置,而 `caesar_decrypt` 函数将字符串中的字符向前移动 `shift` 个位置,从而实现了凯撒密码的加密和解密。
如果要对一个字符串中的字母进行倒排序,可以使用 C 语言的 `qsort` 函数进行排序,具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int cmp(const void *a, const void *b) {
return *(char*)b - *(char*)a;
}
int main() {
char str[] = "hello world";
int len = strlen(str);
qsort(str, len, sizeof(char), cmp);
printf("Sorted string: %s\n", str);
return 0;
}
```
其中,`qsort` 函数接受一个数组、数组长度、每个元素的大小和一个比较函数作为参数,比较函数可以自己实现,这里使用了一个简单的比较函数,将两个字符进行比较,如果第一个字符比第二个字符小,则返回负数,否则返回正数。最后,将得到的排序后的字符串输出即可。
阅读全文
相关推荐
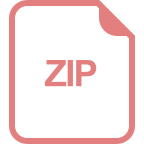
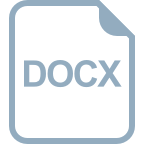














