用c++实现基于openssl的大文件的签名和验证签名
时间: 2023-11-12 19:06:39 浏览: 145
如果要对大文件进行签名,直接读取整个文件会导致内存溢出,因此需要使用流式处理方式,逐块读取文件进行签名。以下是一个示例程序,可以用来对大文件进行签名和验证签名:
```c++
#include <iostream>
#include <fstream>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
using namespace std;
// 生成密钥对
bool generate_key(int bits, string pub_file, string pri_file)
{
RSA *rsa = RSA_new();
BIGNUM *bn = BN_new();
BN_set_word(bn, RSA_F4);
RSA_generate_key_ex(rsa, bits, bn, NULL);
// 保存公钥
FILE *pub_fp = fopen(pub_file.c_str(), "wb");
PEM_write_RSAPublicKey(pub_fp, rsa);
fclose(pub_fp);
// 保存私钥
FILE *pri_fp = fopen(pri_file.c_str(), "wb");
PEM_write_RSAPrivateKey(pri_fp, rsa, NULL, NULL, 0, NULL, NULL);
fclose(pri_fp);
RSA_free(rsa);
BN_free(bn);
return true;
}
// 签名
bool sign(string pri_file, string file, string &signature)
{
RSA *rsa = RSA_new();
// 读取私钥
FILE *fp = fopen(pri_file.c_str(), "rb");
rsa = PEM_read_RSAPrivateKey(fp, NULL, NULL, NULL);
fclose(fp);
// 打开文件
ifstream ifs(file, ios::in | ios::binary);
if (!ifs) {
RSA_free(rsa);
return false;
}
// 初始化SHA256上下文
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256_CTX sha256;
SHA256_Init(&sha256);
// 逐块读取文件并计算SHA256值
char buf[4096];
while (ifs) {
ifs.read(buf, sizeof(buf));
SHA256_Update(&sha256, buf, ifs.gcount());
}
// 计算签名
SHA256_Final(hash, &sha256);
unsigned char sign[RSA_size(rsa)];
unsigned int sign_len;
int ret = RSA_sign(NID_sha256, hash, SHA256_DIGEST_LENGTH, sign, &sign_len, rsa);
if (ret != 1) {
RSA_free(rsa);
return false;
}
signature = string((char *)sign, sign_len);
RSA_free(rsa);
return true;
}
// 验证签名
bool verify(string pub_file, string file, string signature)
{
RSA *rsa = RSA_new();
// 读取公钥
FILE *fp = fopen(pub_file.c_str(), "rb");
rsa = PEM_read_RSAPublicKey(fp, NULL, NULL, NULL);
fclose(fp);
// 打开文件
ifstream ifs(file, ios::in | ios::binary);
if (!ifs) {
RSA_free(rsa);
return false;
}
// 初始化SHA256上下文
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256_CTX sha256;
SHA256_Init(&sha256);
// 逐块读取文件并计算SHA256值
char buf[4096];
while (ifs) {
ifs.read(buf, sizeof(buf));
SHA256_Update(&sha256, buf, ifs.gcount());
}
// 验证签名
int ret = RSA_verify(NID_sha256, hash, SHA256_DIGEST_LENGTH, (unsigned char *)signature.c_str(), signature.size(), rsa);
RSA_free(rsa);
return ret == 1;
}
int main()
{
// 生成密钥对
generate_key(2048, "public.pem", "private.pem");
// 签名和验证签名
string file = "large_file.bin";
string signature;
if (sign("private.pem", file, signature)) {
cout << "signature: " << signature << endl;
if (verify("public.pem", file, signature)) {
cout << "verify success" << endl;
} else {
cout << "verify fail" << endl;
}
} else {
cout << "sign fail" << endl;
}
return 0;
}
```
这个程序逐块读取文件进行签名和验证签名,可以处理大文件。你可以根据自己的需要修改程序,比如可以使用其他加密算法、调整块大小等。
阅读全文
相关推荐
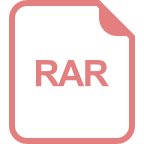
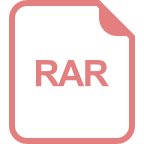
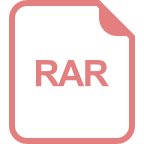















