C语言写一段代码,找出一个数组中出现最多的数,若是出现次数一样多,则输出最小的数
时间: 2023-09-03 15:08:38 浏览: 44
以下是一段C语言代码,实现了找出一个数组中出现最多的数,若是出现次数一样多,则输出最小的数的功能:
```c
#include <stdio.h>
#define MAX_SIZE 100
int main() {
int arr[MAX_SIZE], freq[MAX_SIZE];
int n, i, j, max_freq, min_num;
printf("Enter the size of the array: ");
scanf("%d", &n);
printf("Enter the elements of the array:\n");
for (i = 0; i < n; i++) {
scanf("%d", &arr[i]);
freq[i] = -1;
}
for (i = 0; i < n; i++) {
int count = 1;
for (j = i + 1; j < n; j++) {
if (arr[i] == arr[j]) {
count++;
freq[j] = 0;
}
}
if (freq[i] != 0) {
freq[i] = count;
}
}
max_freq = freq[0];
min_num = arr[0];
for (i = 1; i < n; i++) {
if (freq[i] > max_freq) {
max_freq = freq[i];
min_num = arr[i];
} else if (freq[i] == max_freq) {
if (arr[i] < min_num) {
min_num = arr[i];
}
}
}
printf("The most frequent number is %d\n", min_num);
return 0;
}
```
首先,我们定义了一个数组 `arr` 来存储输入的数,以及一个数组 `freq` 来存储每个数出现的次数。我们先将 `freq` 数组的所有元素初始化为 -1,表示还没有统计过该数的出现次数。
然后,我们使用两个循环来统计每个数出现的次数。外层循环遍历数组中的每个数,内层循环从该数的下一个位置开始遍历,如果有相同的数,则将该数的出现次数加 1,并将 `freq` 数组中对应位置的值设为 0 表示已经统计过该数的出现次数。这样,最后 `freq` 数组中不为 0 的元素就是每个数出现的次数。
接着,我们遍历 `freq` 数组,找到出现次数最多的数,并记录下其出现次数和数值。如果有多个数出现次数相同,则取其中数值最小的数作为结果。
最后,我们输出结果即可。
相关推荐
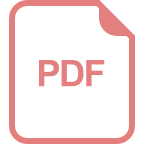
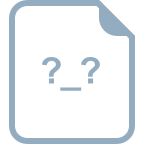
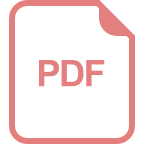














