Python实现手势控制tello无人机的代码
时间: 2024-05-03 17:23:29 浏览: 198
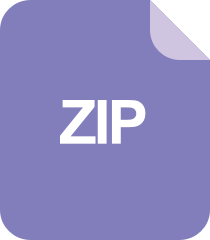
PythonDroneCode:控制Tello无人机的Python代码

此代码需要安装tellopy库和opencv-python库。
```python
import time
import cv2
import tellopy
# 定义手势识别函数
def gesture_recognition():
# 打开电脑摄像头
cap = cv2.VideoCapture(0)
# 读取手势识别模型
gesture = cv2.CascadeClassifier("gesture.xml")
while True:
# 读取摄像头中的图像
ret, frame = cap.read()
# 灰度化处理
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 进行手势检测
hands = gesture.detectMultiScale(gray, 1.3, 5)
# 绘制矩形框
for (x, y, w, h) in hands:
cv2.rectangle(frame, (x, y), (x + w, y + h), (255, 0, 0), 2)
# 显示图像
cv2.imshow("gesture recognition", frame)
# 按下q键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
# 定义tello无人机控制函数
def tello_control():
# 创建tello对象
drone = tellopy.Tello()
# 连接tello无人机
drone.connect()
# 启动视频流传输
drone.start_video()
# 等待无人机准备就绪
time.sleep(1)
# 打开电脑摄像头
cap = cv2.VideoCapture(0)
# 读取手势识别模型
gesture = cv2.CascadeClassifier("gesture.xml")
# 设置飞行方向
direction = ""
while True:
# 读取摄像头中的图像
ret, frame = cap.read()
# 灰度化处理
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 进行手势检测
hands = gesture.detectMultiScale(gray, 1.3, 5)
# 绘制矩形框
for (x, y, w, h) in hands:
cv2.rectangle(frame, (x, y), (x + w, y + h), (255, 0, 0), 2)
# 判断手势
if w > h:
direction = "left" if x < 200 else "right" if x > 400 else ""
else:
direction = "up" if y < 150 else "down" if y > 350 else ""
# 显示图像
cv2.imshow("tello control", frame)
# 按下q键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 控制tello无人机飞行
if direction == "left":
drone.left(30)
elif direction == "right":
drone.right(30)
elif direction == "up":
drone.up(30)
elif direction == "down":
drone.down(30)
else:
drone.hover()
# 停止视频流传输
drone.stop_video()
# 断开连接
drone.disconnect()
# 释放资源
cap.release()
cv2.destroyAllWindows()
if __name__ == '__main__':
# 执行手势识别函数
# gesture_recognition()
# 执行tello无人机控制函数
tello_control()
```
在代码中,手势识别函数使用OpenCV库进行手势检测,识别手势后显示在电脑屏幕上,通过判断手势的方向,将其转换为对tello无人机的控制指令。
tello无人机控制函数通过连接tello无人机,启动视频流传输,打开电脑摄像头,读取手势识别模型,检测手势方向,并将其转换为对tello无人机的控制指令。最后,停止视频流传输,断开连接,释放资源。
阅读全文
相关推荐
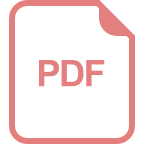
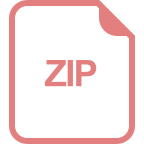
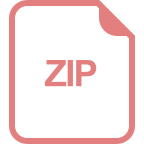
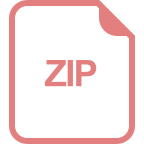
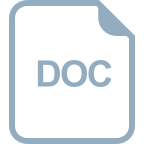
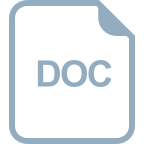
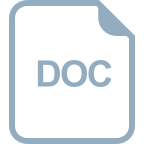
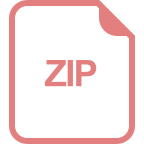
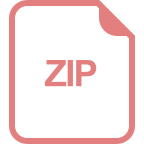
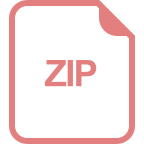
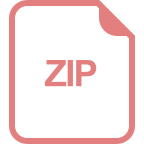
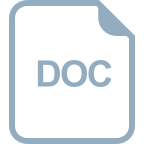




