转换成python代码void GameDraw() { BeginBatchDraw(); //双缓冲防止画面闪烁卡顿 cleardevice(); //刷新界面 for (int i = 0; i < ROW; i++) for (int j = 0; j < COL; j++) outtextxy(j*SIZE,i*SIZE, text[map[i][j]]); //输出在界面 坐标 x-行 y-列 EndBatchDraw(); }
时间: 2024-01-13 14:05:54 浏览: 86
以下是将该函数转换成Python代码的示例:
```python
import easygraphics as eg
def game_draw():
eg.begin_batch_draw() # 双缓冲防止画面闪烁卡顿
eg.clear_device() # 刷新界面
for i in range(ROW):
for j in range(COL):
eg.outtextxy(j*SIZE, i*SIZE, text[map[i][j]]) # 输出在界面 坐标 x-行 y-列
eg.end_batch_draw()
```
需要注意的是,由于该示例代码没有提供函数中所需的全局变量 ROW、COL、SIZE、text 和 map 的定义和初始化,因此需要根据实际情况进行修改。同时,还需要在程序开始处导入 easygraphics 库。
相关问题
easyx双缓冲绘图 void BeginBatchDraw(); void FlushBatchDraw()怎么用
EasyX提供了双缓冲绘图的两个函数BeginBatchDraw()和FlushBatchDraw(),使用这两个函数可以简化双缓冲绘图的实现过程。
BeginBatchDraw()函数用于开启批量绘图模式,该模式下所有的绘图操作都被缓存到内存中,不会立即显示在屏幕上。
FlushBatchDraw()函数用于将缓存的绘图操作刷新到屏幕上,从而显示出来。
具体使用方法如下:
```c++
#include <graphics.h>
int main()
{
initgraph(640, 480); // 初始化图形界面
BeginBatchDraw(); // 开启批量绘图模式
// 在缓存中进行绘图操作
setbkcolor(WHITE);
cleardevice();
settextcolor(BLACK);
outtextxy(100, 100, "Hello, world!");
FlushBatchDraw(); // 将缓存中的绘图操作刷新到屏幕上
// 等待用户按下任意键
getch();
closegraph(); // 关闭图形界面
return 0;
}
```
在上面的代码中,我们使用BeginBatchDraw()函数开启批量绘图模式,然后在缓存中进行绘图操作,最后使用FlushBatchDraw()函数将缓存中的绘图操作刷新到屏幕上。注意,在使用BeginBatchDraw()和FlushBatchDraw()函数时,不需要使用setvisualpage()和setactivepage()函数进行页面的切换,EasyX会自动帮我们完成这个过程。
使用BeginBatchDraw()和FlushBatchDraw()函数可以让双缓冲绘图的实现更加简单和方便。
如何结束#include <stdio.h> #include <graphics.h> #include<time.h> clock_t Start_time; clock_t End_time; int time_1; char str[100]; int main() { initgraph(200, 200, SHOWCONSOLE);//init 初始化 graph 图像窗口 SHOWCONSOLE 显示控制台 setbkcolor(RGB(225, 115, 100));//设置背景颜色 BeginBatchDraw(); //双缓冲绘图,防止屏闪 Start_time = clock(); while (1) { cleardevice(); End_time = clock(); time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC); settextstyle(20, 0, "宋体"); setcolor(BLACK);// sprintf_s(str, " %d s", time_1); outtextxy(10, 10, str); EndBatchDraw();//双缓冲绘图 } return 0; }
这段代码本身没有错误,可以正常运行。但是由于 while 循环中没有任何退出条件,所以程序会一直运行,无法结束。因此你需要添加一个退出循环的条件,以便程序可以正常结束。
下面是一个修改后的代码,添加了一个 ESC 键退出循环的条件:
```c++
#include <stdio.h>
#include <graphics.h>
#include <conio.h>
#include <time.h>
clock_t Start_time;
clock_t End_time;
int time_1;
char str[100];
int main() {
initgraph(200, 200, SHOWCONSOLE); // init 初始化 graph 图像窗口 SHOWCONSOLE 显示控制台
setbkcolor(RGB(225, 115, 100)); // 设置背景颜色
BeginBatchDraw(); // 双缓冲绘图,防止屏闪
Start_time = clock();
while (1) {
cleardevice();
End_time = clock();
time_1 = (int)((End_time - Start_time) / CLOCKS_PER_SEC);
settextstyle(20, 0, "宋体");
setcolor(BLACK);
sprintf_s(str, " %d s", time_1);
outtextxy(10, 10, str);
EndBatchDraw(); // 双缓冲绘图
if (_kbhit() && _getch() == 27) { // 如果按下 ESC 键,则退出循环
break;
}
}
getch();
closegraph();
return 0;
}
```
在这个修改后的代码中,使用 _kbhit 函数检测是否有键盘输入,如果有,则使用 _getch 函数获取键盘输入的字符。如果获取到的字符是 ESC 键,则退出循环,程序正常结束。
阅读全文
相关推荐
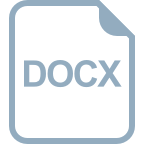
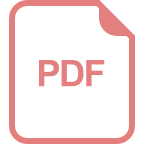
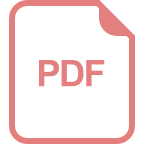










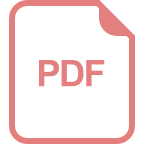
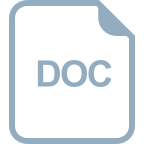
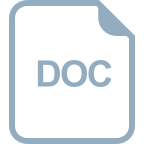
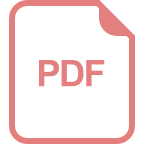