这段代码运行结果是什么:#include <iostream> #include <vector> #include <queue> using namespace std; struct Process { int id; // 进程ID int arrival_time; // 到达时间 int execution_time; // 执行时间 int start_time; // 开始执行时间 int end_time; // 结束执行时间 }; int main() { int n = 15; // 进程数量 int time_slice = 1; // 时间片长度 int current_time = 0; // 当前时间 int total_execution_time = 0; // 总执行时间 int total_wait_time = 0; // 总等待时间 queue<Process> ready_queue; // 就绪队列 // 生成进程 vector<Process> processes(n); for (int i = 0; i < n; i++) { processes[i].id = i + 1; processes[i].arrival_time = rand() % 10; processes[i].execution_time = rand() % 10 + 1; total_execution_time += processes[i].execution_time; } // 模拟轮转算法进行进程调度 while (!ready_queue.empty() || current_time < total_execution_time) { // 将到达时间小于等于当前时间的进程加入就绪队列 for (int i = 0; i < n; i++) { if (processes[i].arrival_time <= current_time && processes[i].execution_time > 0) { ready_queue.push(processes[i]); processes[i].start_time = -1; // 标记为已加入队列 } } // 从就绪队列中选取一个进程执行 if (!ready_queue.empty()) { Process p = ready_queue.front(); ready_queue.pop(); if (p.start_time == -1) { p.start_time = current_time; } if (p.execution_time > time_slice) { current_time += time_slice; p.execution_time -= time_slice; ready_queue.push(p); } else { current_time += p.execution_time; p.execution_time = 0; p.end_time = current_time; total_wait_time += p.start_time - p.arrival_time; cout << "Process " << p.id << ": arrival time = " << p.arrival_time << ", execution time = " << p.execution_time << ", start time = " << p.start_time << ", end time = " << p.end_time << endl; } } } // 输出平均等待时间 double average_wait_time = (double)total_wait_time / n; cout << "Average wait time = " << average_wait_time << endl; return 0; }
时间: 2024-03-18 11:44:10 浏览: 21
这段代码模拟了一个进程调度的过程,采用了轮转算法。程序会首先生成一些进程,然后按照到达时间把它们加入就绪队列中,然后每次从就绪队列中选取一个进程进行执行,如果该进程的执行时间超过了一个时间片长度,那么就把它重新加入就绪队列中,否则就执行完毕并输出该进程的信息。最后输出平均等待时间。
具体输出结果需要运行代码才能得到,因为进程的生成是随机的。
相关问题
先来先服务进程调度c++代码
以下是一个简单的先来先服务进程调度的C++代码:
```c++
#include <iostream>
#include <queue>
using namespace std;
struct Process {
int pid; // 进程ID
int arrival_time; // 到达时间
int burst_time; // 执行时间
};
bool operator<(const Process& p1, const Process& p2) {
return p1.arrival_time > p2.arrival_time;
}
void FCFS(vector<Process>& processes) {
priority_queue<Process> pq;
int current_time = 0;
double total_waiting_time = 0;
for (int i = 0; i < processes.size(); i++) {
pq.push(processes[i]);
}
while (!pq.empty()) {
Process p = pq.top();
pq.pop();
current_time += p.burst_time;
total_waiting_time += current_time - p.arrival_time - p.burst_time;
}
cout << "平均等待时间:" << total_waiting_time / processes.size() << endl;
}
int main() {
vector<Process> processes = {{1, 0, 10}, {2, 3, 5}, {3, 5, 8}, {4, 7, 3}};
FCFS(processes);
return 0;
}
```
进程调度队列排序的C++的代码
以下是一个简单的进程调度队列排序的C++代码示例:
```c++
#include <iostream>
#include <queue>
#include <string>
#include <algorithm>
using namespace std;
// 进程结构体
struct Process {
string name; // 进程名
int priority; // 优先级
int burstTime; // 执行时间
};
// 定义比较函数,用于优先队列排序
struct CompareProcess {
bool operator()(const Process& p1, const Process& p2) {
return p1.priority < p2.priority;
}
};
int main() {
// 创建一个优先队列,用于存放进程
priority_queue<Process, vector<Process>, CompareProcess> pq;
// 添加进程
pq.push({"P1", 3, 5});
pq.push({"P2", 1, 8});
pq.push({"P3", 2, 3});
pq.push({"P4", 4, 2});
// 遍历队列,输出进程信息
while(!pq.empty()) {
Process p = pq.top();
cout << "Process " << p.name << " (Priority: " << p.priority << ", Burst Time: " << p.burstTime << ")" << endl;
pq.pop();
}
return 0;
}
```
在上面的代码中,我们首先定义了一个进程结构体 `Process`,包含进程名称、优先级和执行时间。然后,我们定义了一个比较函数 `CompareProcess`,用于将进程按照优先级从小到大排序,这个函数将作为优先队列的第三个参数传入。
在 `main` 函数中,我们创建了一个优先队列 `pq`,并添加了四个进程。然后,我们使用 `while` 循环遍历队列,每次取出队列头部的进程,并输出其信息。
运行以上代码,输出结果如下:
```
Process P4 (Priority: 4, Burst Time: 2)
Process P1 (Priority: 3, Burst Time: 5)
Process P3 (Priority: 2, Burst Time: 3)
Process P2 (Priority: 1, Burst Time: 8)
```
可以看到,输出的进程按照优先级从小到大排序,符合我们的预期。
相关推荐
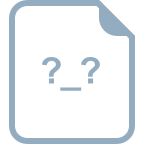












