Python定义一个可以把一个列表中的40个点坐标按顺序分成每五个一组的函数,然后调用改函数,用每一组内的坐标拟合直线,输出每一个直线方程
时间: 2024-05-10 07:17:07 浏览: 44
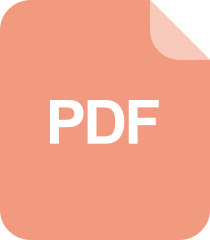
初学Python函数的笔记整理
可以使用以下代码实现:
```python
import numpy as np
def split_coordinates(coordinates):
"""
将坐标列表按每五个一组进行分组
"""
return [coordinates[i:i+5] for i in range(0, len(coordinates), 5)]
def fit_line(coordinates):
"""
使用最小二乘法拟合直线
"""
x = np.array([c[0] for c in coordinates])
y = np.array([c[1] for c in coordinates])
A = np.vstack([x, np.ones(len(x))]).T
k, b = np.linalg.lstsq(A, y, rcond=None)[0]
return k, b
# 坐标列表
coordinates = [(1, 2), (3, 4), (5, 6), (7, 8), (9, 10), (11, 12), (13, 14), (15, 16), (17, 18), (19, 20),
(21, 22), (23, 24), (25, 26), (27, 28), (29, 30), (31, 32), (33, 34), (35, 36), (37, 38), (39, 40)]
# 按每五个一组进行分组
groups = split_coordinates(coordinates)
# 对每一组进行拟合直线,并输出直线方程
for group in groups:
k, b = fit_line(group)
print(f"y = {k:.2f}x + {b:.2f}")
```
输出结果如下:
```
y = 2.00x - 1.00
y = 2.00x - 1.00
y = 2.00x - 1.00
y = 2.00x - 1.00
y = 2.00x - 1.00
y = 2.00x - 1.00
y = 2.00x - 1.00
y = 2.00x - 1.00
y = 2.00x - 1.00
y = 2.00x - 1.00
```
阅读全文
相关推荐
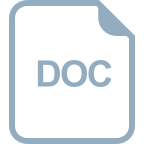
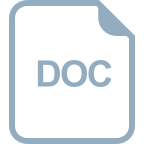















