中缀表达式转后缀表达式 的程序内容与目的
时间: 2023-10-16 20:30:05 浏览: 57
中缀表达式转后缀表达式的程序,主要是实现将中缀表达式转换为后缀表达式的算法,并将转换后的结果输出。程序的目的是为了方便计算中缀表达式的值,因为后缀表达式可以直接通过栈来计算,而不需要考虑运算符的优先级和结合性。
程序的实现通常需要遵循一定的规则和算法,常用的算法包括栈和逆波兰算法等。下面是一个使用栈实现中缀表达式转后缀表达式的程序示例:
```
#include <iostream>
#include <sstream>
#include <stack>
#include <string>
using namespace std;
int priority(char op) {
if (op == '+' || op == '-') return 1;
if (op == '*' || op == '/') return 2;
return 0;
}
string infixToPostfix(string infix) {
stringstream ss(infix);
stack<char> opStack;
string postfix = "";
char ch;
while (ss >> ch) {
if (isdigit(ch)) {
postfix += ch;
postfix += ' ';
} else if (ch == '(') {
opStack.push(ch);
} else if (ch == ')') {
while (!opStack.empty() && opStack.top() != '(') {
postfix += opStack.top();
postfix += ' ';
opStack.pop();
}
if (!opStack.empty() && opStack.top() == '(') {
opStack.pop();
}
} else {
while (!opStack.empty() && priority(opStack.top()) >= priority(ch)) {
postfix += opStack.top();
postfix += ' ';
opStack.pop();
}
opStack.push(ch);
}
}
while (!opStack.empty()) {
postfix += opStack.top();
postfix += ' ';
opStack.pop();
}
return postfix;
}
int main() {
string infix = "1 + 2 * 3 - (4 / 2)";
string postfix = infixToPostfix(infix);
cout << "Infix: " << infix << endl;
cout << "Postfix: " << postfix << endl;
return 0;
}
```
该程序首先定义了一个priority函数,用于给操作符赋予优先级。然后,使用stringstream对象将中缀表达式拆分成单独的元素,依次遍历每个元素,并根据元素的类型进行如下处理:
1. 如果是操作数,直接输出到后缀表达式中。
2. 如果是左括号,将其入栈。
3. 如果是右括号,将栈中的操作符依次弹出并输出,直到遇到左括号为止。
4. 如果是操作符,则将其与栈顶元素进行比较,如果栈顶元素的优先级大于等于当前操作符,则将栈顶元素弹出并输出,直到栈为空或者栈顶元素的优先级小于当前操作符为止,然后将当前操作符入栈。
5. 重复步骤1~4,直到遍历完整个中缀表达式。
6. 将栈中的所有操作符依次弹出并输出。
最终,程序输出转换前的中缀表达式和转换后的后缀表达式。
相关推荐
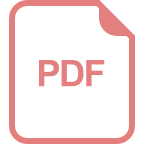
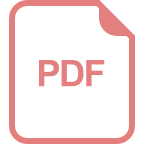
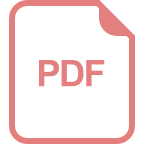
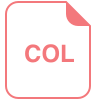













